How to Build a Spring Boot Application
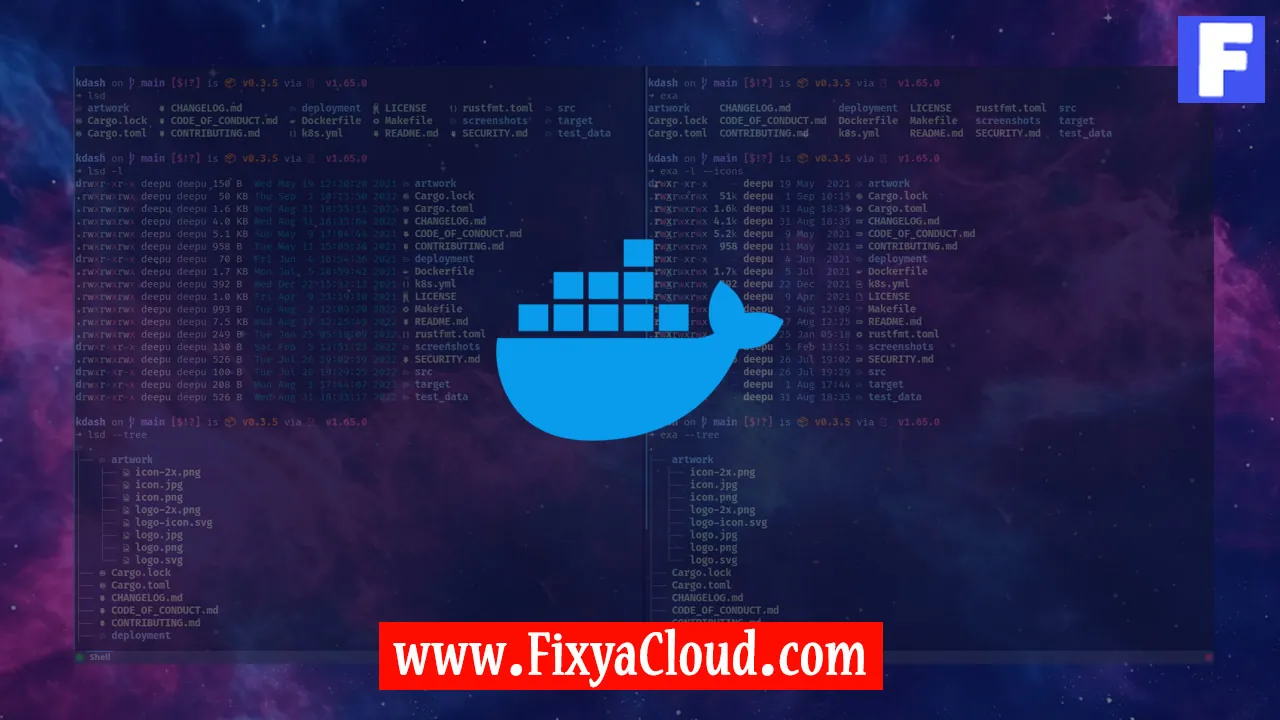
Building a Spring Boot application can be a rewarding journey, providing you with a robust foundation for developing Java-based web applications quickly and efficiently. In this comprehensive guide, we will walk you through the process step by step, from setting up your development environment to deploying your application. Whether you're a seasoned developer or just getting started, this tutorial will help you navigate the world of Spring Boot with ease.
Prerequisites:
Before diving into the tutorial, ensure that you have the following prerequisites installed on your machine:
Java Development Kit (JDK): Make sure you have Java installed on your system. You can download it from the official Oracle website or use OpenJDK.
Integrated Development Environment (IDE): Choose an IDE of your preference, such as IntelliJ IDEA or Eclipse. These IDEs offer excellent support for Spring Boot development.
Setting Up Your Project:
Create a New Spring Boot Project:
Open your IDE and create a new Spring Boot project. Most modern IDEs provide a wizard to guide you through this process. Ensure that you include the necessary dependencies, such as Spring Web, in your project setup.Project Structure:
Familiarize yourself with the project structure. Spring Boot follows a convention-over-configuration approach, so understanding the default structure will make your development smoother.
Building Your First Controller:
- Create a Controller Class:
Build a simple controller class to handle incoming HTTP requests. Annotate it with@RestController
to let Spring know that this class will handle web requests.
@RestController
public class HelloWorldController {
@GetMapping("/hello")
public String sayHello() {
return "Hello, Spring Boot!";
}
}
- Run Your Application:
Use your IDE to run the Spring Boot application. Navigate tohttp://localhost:8080/hello
in your web browser to see the "Hello, Spring Boot!" message.
Working with Data:
Define a Model:
Create a simple model class representing an entity in your application. Annotate it with@Entity
if you plan to persist it in a database.Repository and Service:
Implement a repository interface and a service class to manage data operations. Use annotations like@Repository
and@Service
to facilitate Spring's dependency injection.
Adding Frontend Components:
Thymeleaf Templates:
Integrate Thymeleaf as your template engine for server-side rendering. Create HTML templates in thesrc/main/resources/templates
directory.Controller for Views:
Modify your controller to return the appropriate view names, allowing Thymeleaf to render the HTML templates.
Testing Your Application:
Unit Testing:
Write unit tests for your controllers, services, and repositories. Use tools like JUnit and Mockito to ensure the reliability of your code.Integration Testing:
Perform integration tests to validate the interactions between different components of your Spring Boot application.
Deployment:
Create a JAR or WAR file:
Use Maven or Gradle to build a deployable JAR or WAR file for your Spring Boot application.Deploy to a Server:
Deploy your application to a server of your choice. Popular choices include Apache Tomcat or deploying as a standalone JAR.
Congratulations! You've successfully built a Spring Boot application from scratch, incorporating controllers, data handling, and even frontend components. This tutorial has provided you with a solid foundation, but there's always more to explore and learn in the dynamic world of Spring Boot development.
Related Searches and Questions asked:
That's it for this topic, Hope this article is useful. Thanks for Visiting us.