How to Run Spring Boot on Docker
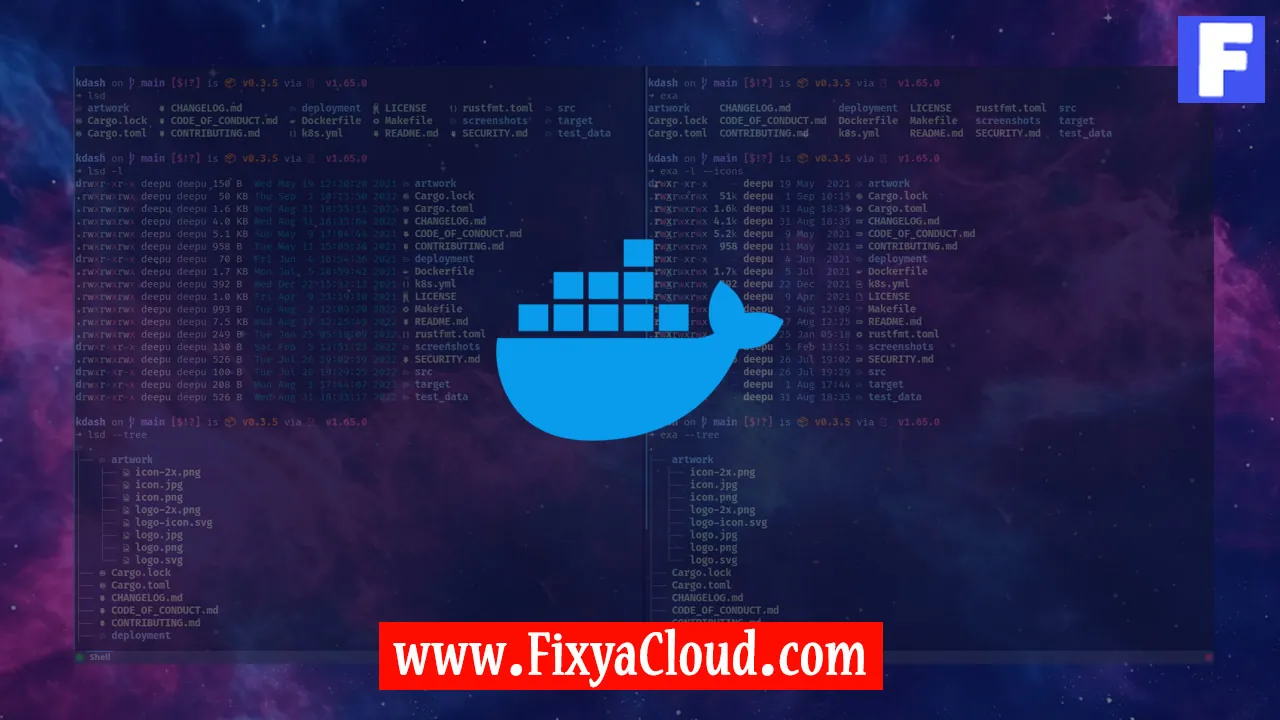
In the ever-evolving landscape of software development, containerization has become a crucial aspect of building, shipping, and deploying applications. Docker, a popular containerization platform, allows developers to encapsulate applications and their dependencies into lightweight containers. When combined with Spring Boot, a powerful Java-based framework for building microservices, you can achieve seamless deployment and scaling. This article will guide you through the process of running a Spring Boot application on Docker, ensuring a smooth and efficient development and deployment workflow.
Prerequisites:
Before diving into containerizing your Spring Boot application, ensure that you have Docker installed on your machine. Additionally, make sure you have a Spring Boot application ready to go.Dockerizing Your Spring Boot Application:
The first step is to create a Docker image for your Spring Boot application. To do this, you'll need a Dockerfile in the root of your project. Here's a basic example:# Use an official OpenJDK runtime as a parent image
FROM openjdk:11-jre-slim
# Set the working directory to /app
WORKDIR /app
# Copy the current directory contents into the container at /app
ADD . /app
# Specify the command to run on container start
CMD ["java", "-jar", "your-spring-boot-app.jar"]Building the Docker Image:
Open a terminal in the directory containing your Dockerfile and run the following command to build your Docker image:docker build -t your-image-name .
Running the Docker Container:
Once the image is built, you can run your Spring Boot application in a Docker container using the following command:docker run -p 8080:8080 your-image-name
This command maps port 8080 on your local machine to port 8080 in the Docker container.
Accessing Your Spring Boot Application:
Open your web browser and navigate tohttp://localhost:8080
to access your Spring Boot application running inside the Docker container.Additional Docker Commands:
- To list running containers:
docker ps
- To stop a running container:
docker stop container-id
- To remove a container:
docker rm container-id
- To remove an image:
docker rmi your-image-name
- To list running containers:
More Examples:
Using Docker Compose:
Create adocker-compose.yml
file to define multi-container Docker applications. This is useful when your Spring Boot application depends on other services.version: '3'
services:
app:
image: your-image-name
ports:
- "8080:8080"Run your application with:
docker-compose up
Environment Variables:
Customize your Spring Boot application's behavior in a container by using environment variables. Modify your Dockerfile or docker-compose.yml file to include environment variables.
Dockerizing your Spring Boot application provides a portable and consistent environment, making it easier to manage dependencies and deploy your application across various environments. Embrace containerization to streamline your development process and enhance the scalability of your Spring Boot applications.
Related Searches and Questions asked:
That's it for this topic, Hope this article is useful. Thanks for Visiting us.