Kubernetes Microservices with Docker
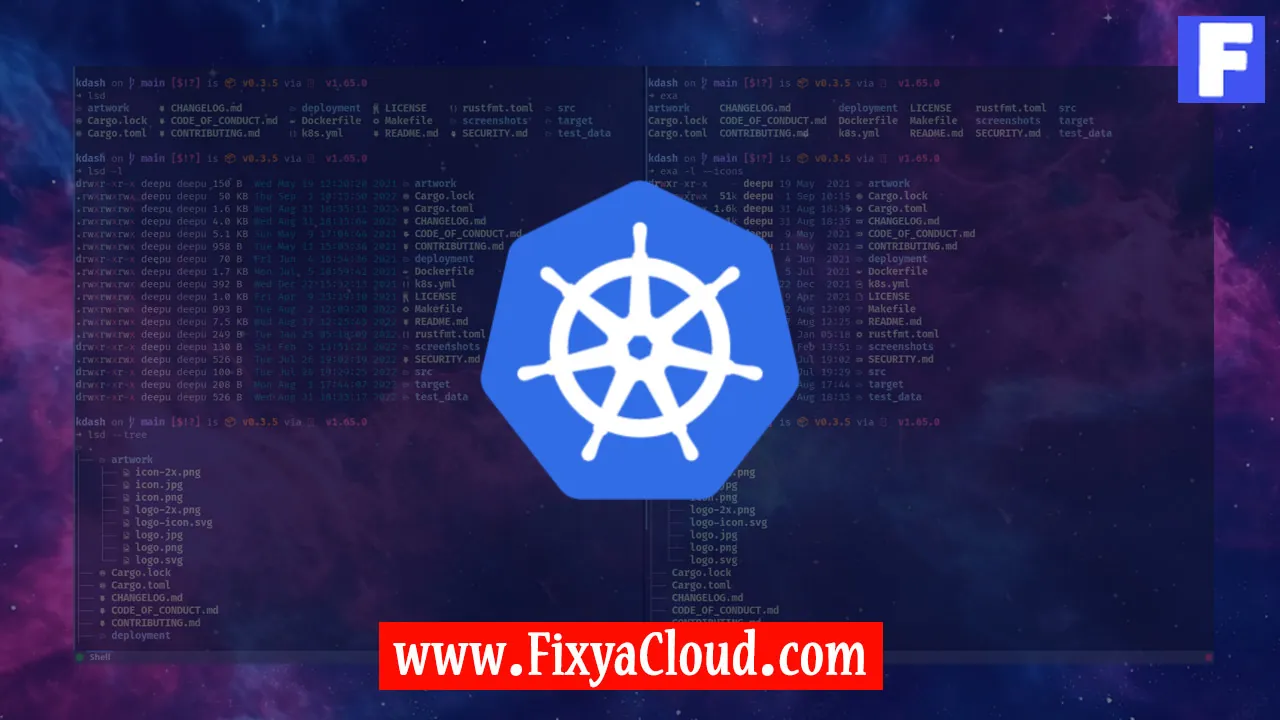
In the ever-evolving landscape of software development, orchestrating microservices efficiently is crucial for seamless deployment and scalability. Kubernetes, coupled with Docker, provides a powerful combination to manage and deploy microservices effectively. In this article, we will explore the synergy between Kubernetes and Docker, unraveling the steps to build and orchestrate microservices.
Understanding Kubernetes and Docker:
Before diving into the technicalities, let's understand the basics. Docker is a containerization platform that enables developers to package applications and their dependencies into a standardized unit, known as a container. On the other hand, Kubernetes is an open-source container orchestration platform that automates the deployment, scaling, and management of containerized applications. Together, they streamline the process of deploying and managing microservices.
Setting Up Docker:
The first step in our journey is to set up Docker. If you haven't installed Docker on your machine, you can do so by following these commands:
# Install Docker on Linux
sudo apt-get update
sudo apt-get install docker-ce
# Install Docker on macOS
brew install docker
# Install Docker on Windows
Download and run the Docker Desktop installer from the official website.
Ensure Docker is running by executing:
docker --version
docker run hello-world
Creating Microservices with Docker:
Now that Docker is up and running, let's create a simple microservice. Create a file named app.py
with the following Python code:
# app.py
from flask import Flask
app = Flask(__name__)
@app.route('/')
def hello():
return "Hello, this is my microservice!"
Next, create a Dockerfile
to define the Docker image:
# Dockerfile
FROM python:3.8-slim
COPY requirements.txt /app/requirements.txt
WORKDIR /app
RUN pip install -r requirements.txt
COPY . /app
CMD ["python", "app.py"]
Build the Docker image:
docker build -t my-microservice:1.0 .
Run the microservice:
docker run -p 5000:5000 my-microservice:1.0
Access the microservice in your browser at http://localhost:5000.
Orchestrating Microservices with Kubernetes:
Now, let's move on to orchestrating our microservices using Kubernetes.
Ensure you have kubectl
(Kubernetes command-line tool) installed:
# Install kubectl on Linux
sudo apt-get update && sudo apt-get install -y kubectl
# Install kubectl on macOS
brew install kubectl
# Install kubectl on Windows
Download kubectl from the official website.
Deploying Microservices with Kubernetes:
Create a Kubernetes deployment YAML file named microservice-deployment.yaml
:
# microservice-deployment.yaml
apiVersion: apps/v1
kind: Deployment
metadata:
name: my-microservice
spec:
replicas: 2
selector:
matchLabels:
app: my-microservice
template:
metadata:
labels:
app: my-microservice
spec:
containers:
- name: my-microservice
image: my-microservice:1.0
ports:
- containerPort: 5000
Deploy the microservice to Kubernetes:
kubectl apply -f microservice-deployment.yaml
Check the status of the deployment:
kubectl get deployments
kubectl get pods
Scaling Microservices:
One of the key benefits of Kubernetes is the ability to scale applications effortlessly. To scale our microservice, run:
kubectl scale deployment my-microservice --replicas=3
Verify the scaling:
kubectl get pods
In this article, we've embarked on a journey to explore the integration of Kubernetes and Docker for orchestrating microservices. From setting up Docker to deploying and scaling microservices with Kubernetes, we've covered essential steps to empower you in managing your microservices efficiently. As you continue your exploration, remember that the world of containerization and orchestration is vast, and these tools offer limitless possibilities for scalable and resilient architectures.
Related Searches and Questions asked:
That's it for this topic, Hope this article is useful. Thanks for Visiting us.