Deploying Applications with Ansible Playbooks: A Practical Walkthrough
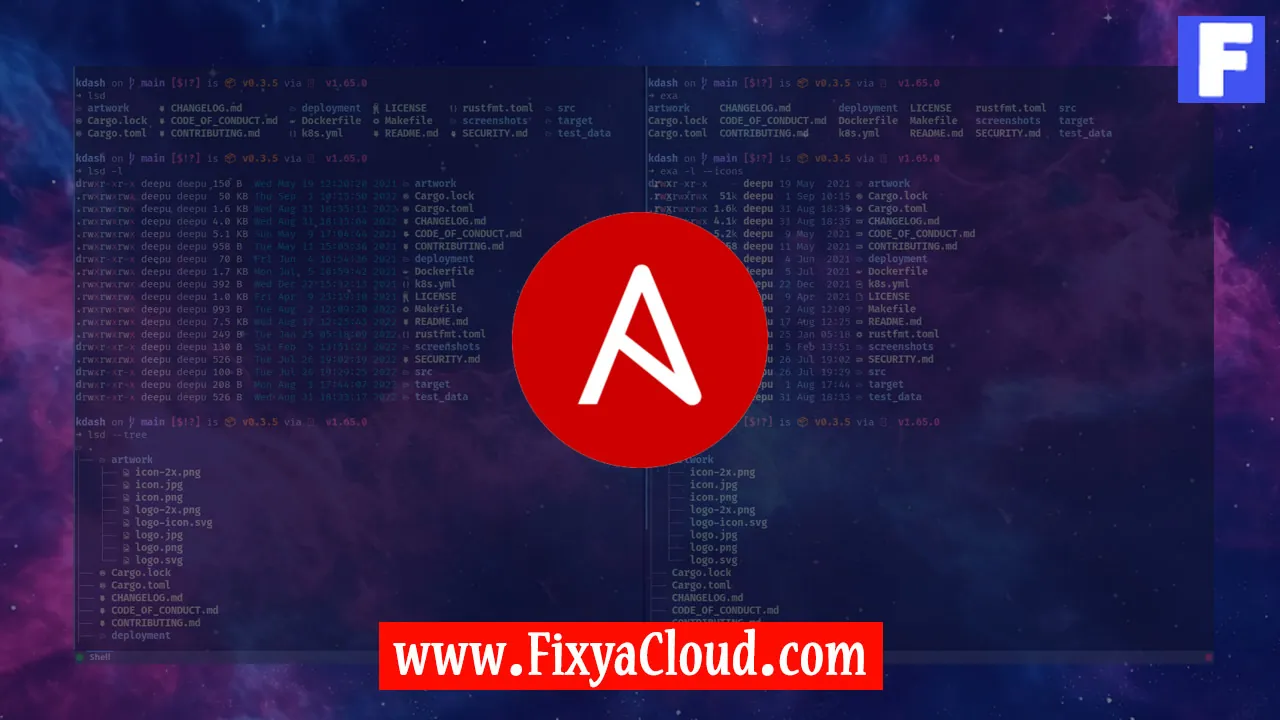
In the ever-evolving landscape of IT and software development, automation has become a crucial component for efficient and error-free deployment processes. Ansible, an open-source automation tool, has gained popularity for its simplicity and effectiveness in managing configurations, orchestrating tasks, and deploying applications. In this article, we will embark on a practical walkthrough of deploying applications using Ansible Playbooks.
Understanding Ansible Playbooks:
Ansible Playbooks are YAML files that define a set of tasks, plays, and roles to be executed on remote servers. They provide a high-level abstraction, making it easier to describe and automate complex deployment processes.Setting Up Your Ansible Environment:
Before diving into playbook creation, ensure that Ansible is installed on your control machine. You can install it using the package manager relevant to your operating system, such as apt for Debian-based systems or yum for Red Hat-based systems.# For Debian-based systems
sudo apt-get update
sudo apt-get install ansible# For Red Hat-based systems
sudo yum install ansibleCreating Your First Ansible Playbook:
Begin by creating a directory for your playbook and navigate into it. Inside, create a file nameddeploy.yml
â this will be your first playbook.# deploy.yml
---
- name: Deploy My Application
hosts: your_target_servers
tasks:
- name: Copy application files
copy:
src: /path/to/your/application
dest: /opt/my_applicationThis simple playbook copies your application files to the specified destination on the target servers.
Executing the Playbook:
To run your playbook, use theansible-playbook
command:ansible-playbook deploy.yml
Ansible will connect to the specified hosts and execute the defined tasks.
Adding Variables and Conditionals:
Enhance your playbook by introducing variables and conditionals. This allows for greater flexibility and adaptability across different environments.# deploy.yml
---
- name: Deploy My Application
hosts: your_target_servers
vars:
app_version: "1.0"
tasks:
- name: Copy application files
copy:
src: /path/to/your/application/{{ app_version }}
dest: /opt/my_applicationIn this example, the
app_version
variable is used to dynamically set the source path.Roles: Organizing Your Playbook:
As your playbook grows, consider organizing it into roles. Roles allow you to modularize your playbook, making it more maintainable and reusable.# site.yml
---
- name: Deploy My Application
hosts: your_target_servers
roles:
- my_applicationCreate a role structure with directories like
roles/my_application/tasks/main.yml
to encapsulate the tasks for deploying your application.Extending Ansible Functionality:
Ansible provides a vast array of modules to extend its functionality. Explore modules for databases, cloud services, and other technologies to integrate seamlessly with your deployment processes.# deploy.yml
---
- name: Deploy Database
hosts: db_servers
tasks:
- name: Install PostgreSQL
apt:
name: postgresql
state: presentThis example shows how to use the
apt
module to install PostgreSQL on database servers.Handling Secrets with Ansible Vault:
Safeguard sensitive information by using Ansible Vault to encrypt variables and files containing sensitive data.ansible-vault create vars.yml
Edit the encrypted file, and then reference it in your playbook:
# deploy.yml
---
- name: Deploy My Application
hosts: your_target_servers
vars_files:
- vars.yml
tasks:
- name: Copy application files
copy:
src: /path/to/your/application
dest: /opt/my_applicationAnsible will prompt you for the vault password during execution.
Scaling and Parallel Execution:
Ansible enables parallel execution of tasks across multiple hosts. Leverage this capability to scale your deployments efficiently.ansible-playbook deploy.yml -f 10
The
-f
option sets the number of parallel forks.
Related Searches and Questions asked:
That's it for this topic, Hope this article is useful. Thanks for Visiting us.