How do I handle errors and exceptions in an Ansible playbook?
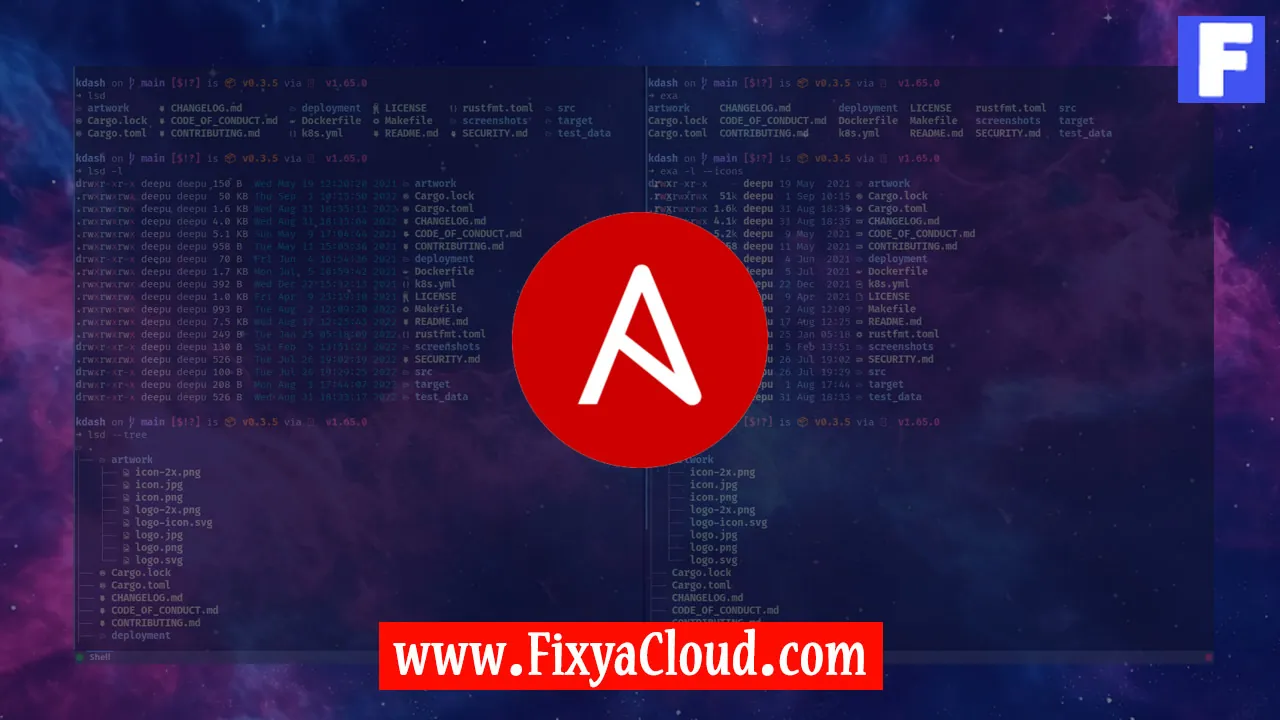
Ansible, an open-source automation tool, simplifies the configuration management and deployment of applications. However, as with any complex system, errors and exceptions are inevitable. Handling these gracefully is crucial to ensure the robustness of your Ansible playbooks. In this article, we will explore various strategies and techniques to effectively manage errors and exceptions in Ansible, providing you with the skills needed to troubleshoot and maintain your automation workflows.
Understanding Ansible Errors and Exceptions:
Ansible provides detailed error messages that can help you identify issues in your playbooks. Before diving into handling errors, it's essential to understand these messages. Use the-vvv
(very verbose) option to get more detailed output, and review Ansible documentation for common error codes.Dealing with Playbook Failures:
To gracefully handle errors within a playbook, use thefailed_when
directive. This allows you to define conditions under which a task should be considered failed. For example:- name: Ensure a file exists
stat:
path: /path/to/file
register: file_status
failed_when: file_status.stat.exists == FalseIgnoring Errors with ignore_errors:
In some cases, you might want to proceed with the playbook execution even if a task fails. Theignore_errors
directive allows you to achieve this. Be cautious when using this option, as it can lead to unintended consequences if not handled properly.- name: Attempt a task that may fail
command: /path/to/failing/command
ignore_errors: yesUsing Blocks for Error Handling:
Ansible 2.10 introduced blocks, providing a structured way to handle errors within a playbook. Blocks allow you to define a set of tasks to be executed, and you can specify a rescue section to handle errors that may occur within the block.- name: Example using blocks for error handling
block:
- name: Task 1
command: /path/to/command1
- name: Task 2
command: /path/to/command2
rescue:
- name: Handle error
debug:
msg: "An error occurred"Error Handling at the Play Level:
You can also handle errors at the play level using theany_errors_fatal
option. When set to true, any task failure within the play will cause the entire play to fail.- name: Playbook with error handling at the play level
hosts: all
any_errors_fatal: true
tasks:
- name: Task 1
command: /path/to/command1
- name: Task 2
command: /path/to/command2Logging and Debugging:
Utilize Ansible's logging and debugging features to gather information about the playbook execution. Thedebug
module and theansible-playbook
command-line options such as-vvv
can provide valuable insights into the execution flow and variable values.- name: Debugging example
debug:
var: some_variableCustom Error Messages with assert:
Theassert
module allows you to define custom conditions that, when not met, will result in a failure. This can be useful for adding descriptive error messages to your playbooks.- name: Ensure a variable is defined
assert:
that:
- my_variable is defined
fail_msg: "my_variable is not defined!"
Effectively handling errors and exceptions is an integral part of creating robust and reliable Ansible playbooks. By understanding the various techniques discussed in this article, you'll be better equipped to troubleshoot, debug, and enhance the resilience of your automation workflows. Remember to regularly review Ansible documentation for updates and additional best practices in error handling.
Related Searches and Questions asked:
That's it for this topic, Hope this article is useful. Thanks for Visiting us.