What are some best practices for managing AWS resources with Ansible?
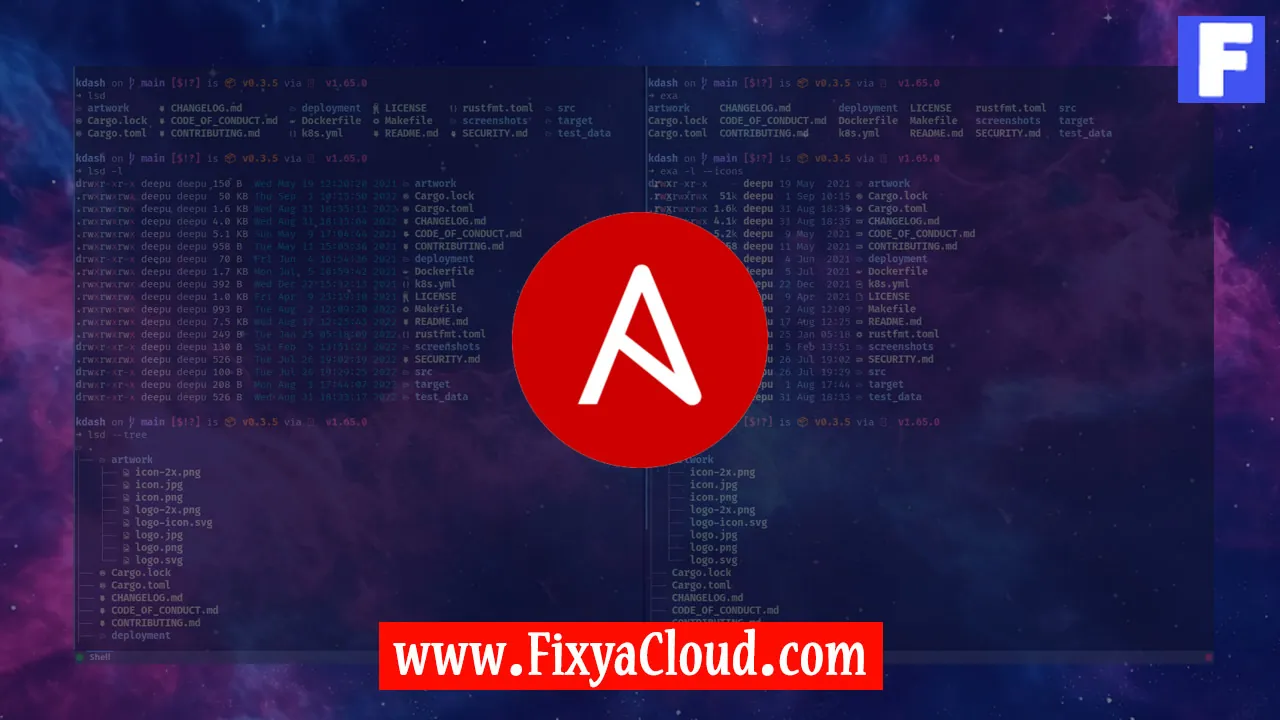
Managing AWS resources efficiently is crucial for organizations leveraging cloud services. Ansible, a powerful automation tool, simplifies this process by allowing users to define and manage infrastructure as code. In this article, we'll explore some best practices for managing AWS resources using Ansible, empowering you to streamline your cloud operations seamlessly.
Setting Up Ansible for AWS:
To begin, ensure Ansible is installed on your system. Use the following commands to install Ansible:sudo apt-get update
sudo apt-get install ansibleOnce installed, configure Ansible with AWS credentials:
export AWS_ACCESS_KEY_ID='your_access_key'
export AWS_SECRET_ACCESS_KEY='your_secret_key'Organizing Ansible Playbooks:
Structure your Ansible playbooks logically for better maintainability. Create separate playbooks for different AWS services or components. This practice makes it easier to manage, troubleshoot, and update your infrastructure code.---
- name: EC2 Instance Provisioning
hosts: localhost
tasks:
- name: Launch EC2 instance
ec2_instance:
key_name: "my-key"
instance_type: "t2.micro"
image_id: "ami-12345678"
count: 1
state: presentUsing Ansible Roles:
Leverage Ansible roles to modularize your playbooks. Roles encapsulate specific functionalities, making your automation code more reusable and readable. Create separate roles for tasks like security group configuration, S3 bucket setup, or IAM role management.---
- name: Include Security Group Role
hosts: localhost
roles:
- security_groupDynamic Inventory Configuration:
Instead of hardcoding IP addresses, utilize dynamic inventory for AWS. Ansible provides an AWS dynamic inventory script that fetches real-time information about your AWS resources.ansible-inventory -i aws_ec2.yaml --list
Tagging for Resource Management:
Tagging is a best practice for organizing and managing AWS resources. Implement tagging in your Ansible playbooks to assign metadata to instances, making it easier to identify, track, and manage resources.- name: Tag EC2 Instances
ec2_tag:
resource: "{{ item.id }}"
tags:
- key: Name
value: "{{ item.tag_name }}"
region: "{{ item.region }}"
loop: "{{ ec2_instance_info.instances }}"Securing AWS Resources:
Implement security best practices using Ansible. Ensure your AWS resources follow security guidelines by configuring IAM roles, securing S3 buckets, and enforcing network security policies.- name: IAM Role Creation
iam_role:
name: "{{ role_name }}"
state: present
policy_document: "{{ lookup('file', 'iam_policy.json') }}"Continuous Integration and Deployment (CI/CD):
Integrate Ansible into your CI/CD pipelines for automated testing and deployment. This ensures that changes to your AWS infrastructure are validated and deployed consistently.stages:
- name: Deploy Infrastructure
jobs:
- name: Provision EC2 Instances
ansible:
playbook: deploy_infrastructure.yaml
Effectively managing AWS resources with Ansible requires a combination of thoughtful organization, modularization, and adherence to best practices. By following the steps and examples outlined in this article, you can harness the full power of Ansible to automate and optimize your AWS infrastructure.
Related Searches and Questions asked:
That's it for this topic, Hope this article is useful. Thanks for Visiting us.