How To Consume an API From a NodeMCU "
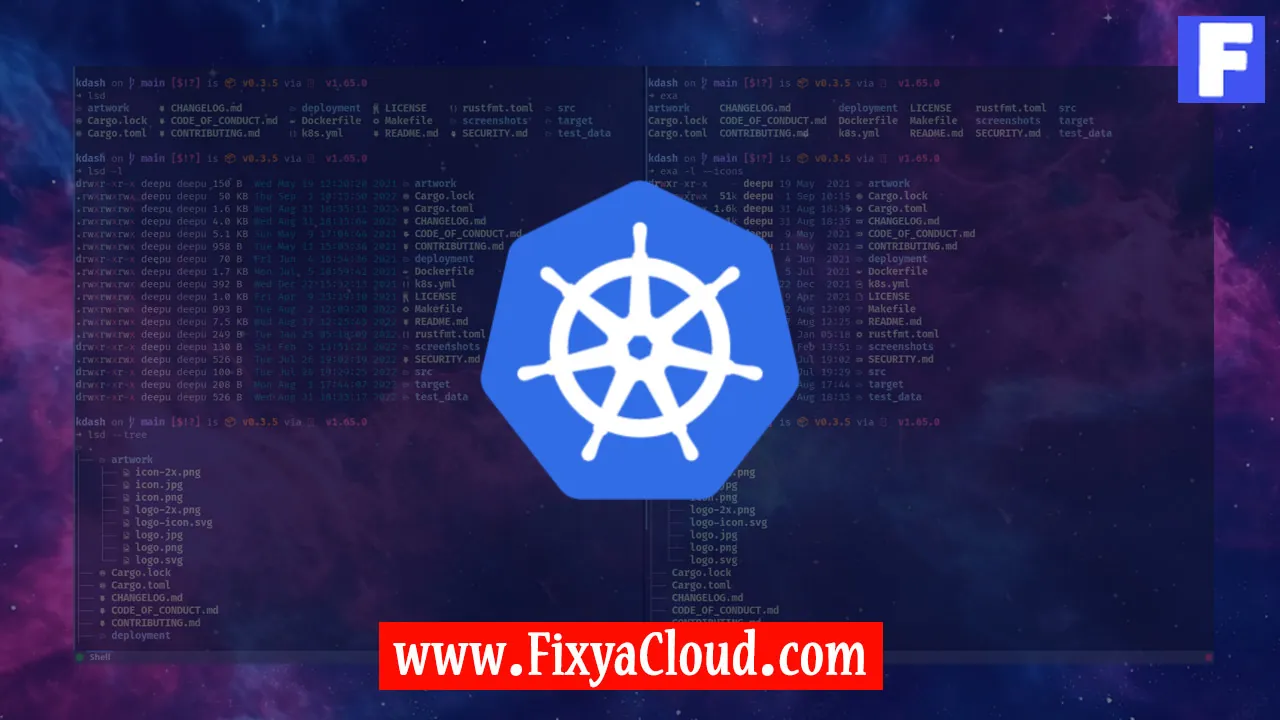
In the ever-evolving landscape of the Internet of Things (IoT), NodeMCU has emerged as a popular platform for building connected devices. One essential aspect of IoT development is the ability to interact with external services, and Application Programming Interfaces (APIs) play a crucial role in facilitating this communication. In this guide, we'll explore the step-by-step process of consuming an API from a NodeMCU, empowering you to integrate your projects with external data and services seamlessly.
Setting Up Your NodeMCU:
Before diving into API consumption, ensure you have your NodeMCU board set up and connected to your development environment. If you haven't done this yet, follow the instructions provided by the NodeMCU documentation.
Understanding APIs:
APIs serve as bridges that allow different software systems to communicate with each other. They define the methods and data formats that applications can use to request and exchange information. In our case, we'll be consuming an API to fetch or send data from our NodeMCU.
Selecting an API:
Choose an API that aligns with your project requirements. Whether it's weather data, stock prices, or any other information, make sure the API you select provides the data you need. Popular choices include OpenWeatherMap, RESTful services, or any other public API that suits your application.
Writing the Code:
Now, let's get our hands dirty with some code. Use the Arduino IDE or your preferred development environment. Start by including the necessary libraries, such as the "ESP8266WiFi.h" library for connecting to Wi-Fi and "HTTPClient.h" for making HTTP requests.
#include <ESP8266WiFi.h>
#include <HTTPClient.h>
const char* ssid = "your-SSID";
const char* password = "your-PASSWORD";
void setup() {
Serial.begin(115200);
WiFi.begin(ssid, password);
while (WiFi.status() != WL_CONNECTED) {
delay(1000);
Serial.println("Connecting to WiFi...");
}
}
void loop() {
// Your API consumption code goes here
}
Replace "your-SSID" and "your-PASSWORD" with your Wi-Fi credentials. This basic setup establishes a Wi-Fi connection, paving the way for API communication.
Making API Requests:
Now, let's make a simple GET request to a sample API. For this example, we'll use the JSONPlaceholder API, a fake online REST API for testing and prototyping.
void loop() {
if (WiFi.status() == WL_CONNECTED) {
HTTPClient http;
http.begin("https://jsonplaceholder.typicode.com/posts/1");
int httpCode = http.GET();
if (httpCode > 0) {
String payload = http.getString();
Serial.println(payload);
}
http.end();
}
delay(5000); // Delay for 5 seconds before making the next request
}
In this snippet, we send a GET request to retrieve information about a post from the JSONPlaceholder API. The response is then printed to the serial monitor.
Handling API Responses:
Depending on the API you're working with, you'll need to parse the response to extract the relevant information. JSON is a common format, and ArduinoJson library can be helpful for parsing JSON data.
#include <ArduinoJson.h>
void loop() {
// ... (previous code)
if (httpCode > 0) {
String payload = http.getString();
const size_t capacity = JSON_OBJECT_SIZE(3) + 90;
DynamicJsonDocument doc(capacity);
deserializeJson(doc, payload);
const char* title = doc["title"];
const char* body = doc["body"];
Serial.print("");
Serial.println(title);
Serial.print("Body: ");
Serial.println(body);
}
// ... (remaining code)
}
This snippet shows how to parse a JSON response and extract specific fields.
Handling POST Requests:
If your application requires sending data to the API, you can modify the code to handle POST requests. For example, sending sensor data to a server.
void loop() {
if (WiFi.status() == WL_CONNECTED) {
HTTPClient http;
http.begin("https://jsonplaceholder.typicode.com/posts");
http.addHeader("Content-Type", "application/json");
// Your JSON payload goes here
String payload = "{\"title\":\"New Post\",\"body\":\"This is the body of the post\",\"userId\":1}";
int httpCode = http.POST(payload);
if (httpCode > 0) {
String response = http.getString();
Serial.println(response);
}
http.end();
}
delay(5000);
}
This example sends a POST request to create a new post on the JSONPlaceholder API.
Testing and Debugging:
As you develop, continually test your code and monitor the serial output for any issues. Debugging is crucial in ensuring smooth communication between your NodeMCU and the API.
Congratulations! You've successfully learned how to consume an API from a NodeMCU. This fundamental skill opens up a world of possibilities for your IoT projects. Experiment with different APIs, explore advanced features, and continue building innovative connected devices.
Related Searches and Questions asked:
That's it for this topic, Hope this article is useful. Thanks for Visiting us.