Deploying a Spring Boot Application with Docker
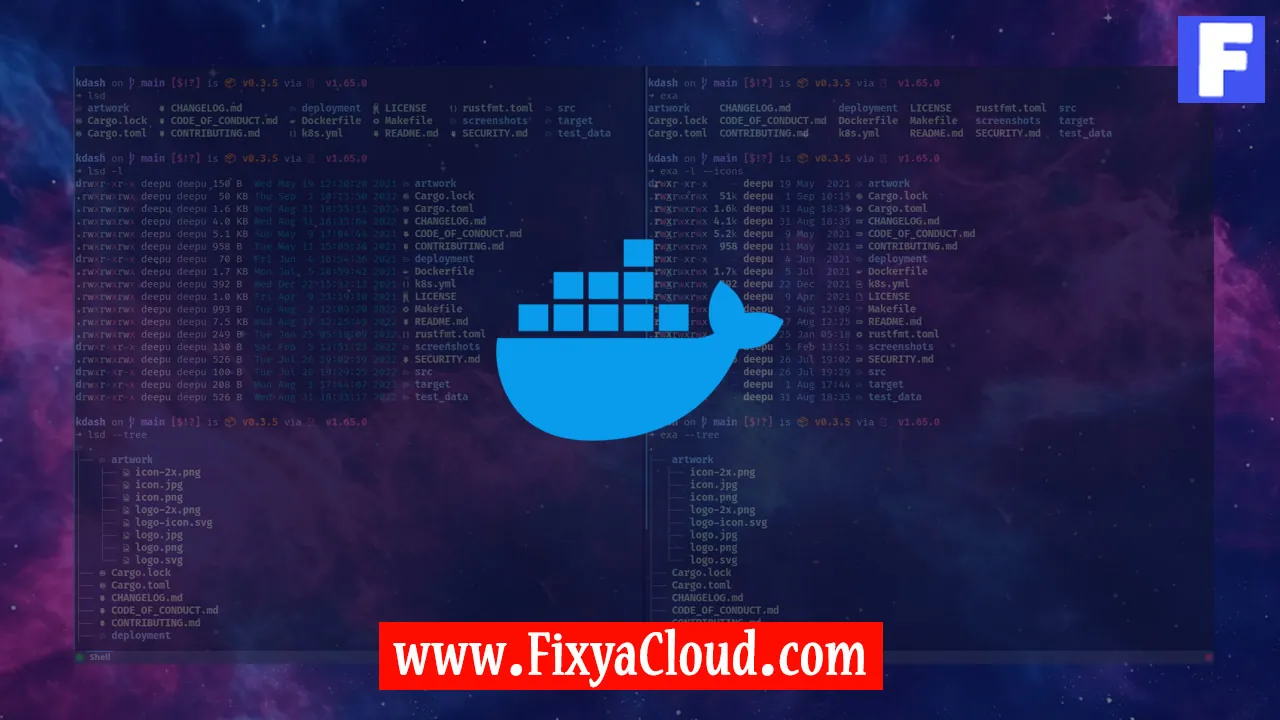
In the dynamic world of software development, deploying applications efficiently is a crucial aspect. Docker has emerged as a game-changer, providing a containerization solution that ensures consistent and reproducible deployments. This article will guide you through the process of deploying a Spring Boot application using Docker, combining the power of Spring Boot's simplicity with Docker's containerization benefits.
Setting the Stage: Prerequisites
Before we dive into deploying our Spring Boot application with Docker, let's ensure you have the necessary tools installed:
Java Development Kit (JDK): Make sure you have the JDK installed on your system.
Spring Boot: Develop your Spring Boot application.
Docker: Install Docker on your machine. You can download it from the official Docker website.
Creating a Spring Boot Application:
Assuming you have a Spring Boot application ready, if not, you can quickly generate one using Spring Initializr.
$ curl https://start.spring.io/starter.zip -o my-spring-boot-app.zip
$ unzip my-spring-boot-app.zip -d my-spring-boot-app
$ cd my-spring-boot-app
Dockerizing the Spring Boot Application:
Now, let's Dockerize our Spring Boot application by creating a Dockerfile in the project root.
# Dockerfile
FROM openjdk:11-jre-slim
COPY target/my-spring-boot-app.jar /app.jar
CMD ["java", "-jar", "/app.jar"]
This Dockerfile uses the official OpenJDK 11 image, copies the JAR file into the container, and sets the command to run the Spring Boot application.
Building the Docker Image:
Navigate to the project directory and build the Docker image.
$ docker build -t my-spring-boot-app .
Running the Docker Container:
Now that we have the Docker image, let's run it in a container.
$ docker run -p 8080:8080 my-spring-boot-app
Your Spring Boot application is now running in a Docker container, accessible at http://localhost:8080.
Additional Docker Commands:
List running containers:
$ docker ps
View container logs:
$ docker logs <container-id>
Stop a running container:
$ docker stop <container-id>
Deploying on Docker Compose:
Docker Compose simplifies the orchestration of multi-container Docker applications. Create a docker-compose.yml
file:
version: '3'
services:
my-spring-boot-app:
image: my-spring-boot-app
ports:
- "8080:8080"
Run your application with:
$ docker-compose up
Congratulations! You've successfully deployed a Spring Boot application with Docker. Containerization provides a consistent and portable environment, making your application deployment hassle-free. Experiment with different Docker features to optimize your development workflow further.
Related Searches and Questions asked:
That's it for this topic, Hope this article is useful. Thanks for Visiting us.