How to Build Java Application with Docker
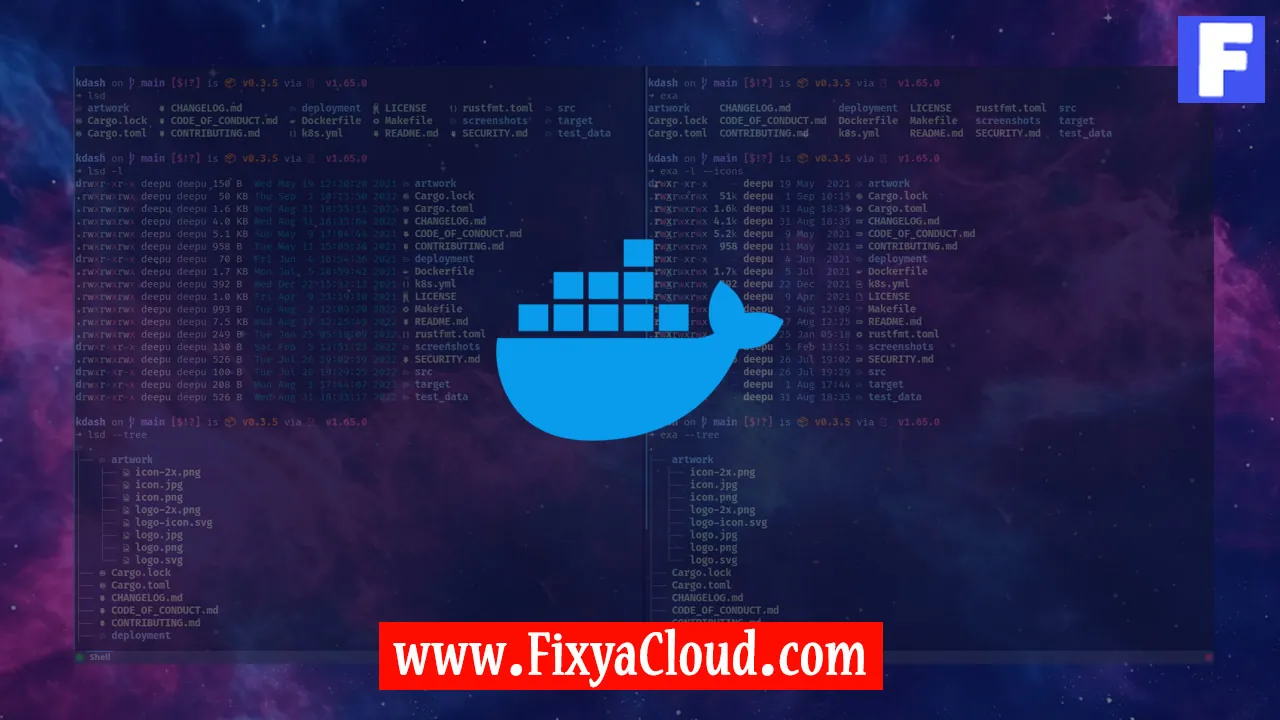
In today's fast-paced software development landscape, efficient deployment and scalability are crucial factors. Docker has emerged as a game-changer, providing a lightweight and portable solution for packaging and deploying applications. This article will guide you through the process of building a Java application using Docker, ensuring seamless integration and hassle-free deployment.
Setting Up Your Development Environment:
Before diving into Docker, make sure you have Java and Docker installed on your machine. You can download Java from the official Oracle website or use OpenJDK. Docker Desktop can be obtained from the official Docker website.Creating a Simple Java Application:
Start by creating a basic Java application. You can use any Integrated Development Environment (IDE) like IntelliJ IDEA or Eclipse. Write a simple "Hello, Docker!" program to demonstrate the application.public class Main {
public static void main(String[] args) {
System.out.println("Hello, Docker!");
}
}Writing a Dockerfile:
The Dockerfile is a crucial component that specifies how your application should be packaged into a Docker image. Create a file namedDockerfile
in your project's root directory.# Use the official OpenJDK base image
FROM openjdk:11
# Set the working directory
WORKDIR /app
# Copy the compiled Java application JAR file
COPY ./target/your-app.jar .
# Specify the command to run your application
CMD ["java", "-jar", "your-app.jar"]Building the Docker Image:
Open a terminal, navigate to your project's directory, and execute the following command to build your Docker image.docker build -t your-app .
Running the Docker Container:
After successfully building the Docker image, you can run a container based on that image.docker run -p 8080:8080 your-app
Adjust the port number according to your application's configuration.
Accessing the Java Application in Docker:
Open your web browser and go tohttp://localhost:8080
. You should see the "Hello, Docker!" message, indicating that your Java application is up and running inside a Docker container.Advanced Docker Commands:
Checking running containers:
docker ps
Viewing container logs:
docker logs <container-id>
Stopping a running container:
docker stop <container-id>
Cleaning up unused resources:
docker system prune -a
In this article, we walked through the process of building a Java application with Docker, from setting up the development environment to running a containerized version of your application. Docker simplifies the deployment process and enhances the scalability of your Java applications. Incorporating Docker into your workflow will undoubtedly contribute to a more efficient and streamlined development process.
Related Searches and Questions asked:
That's it for this topic, Hope this article is useful. Thanks for Visiting us.