Dockerize Java Application
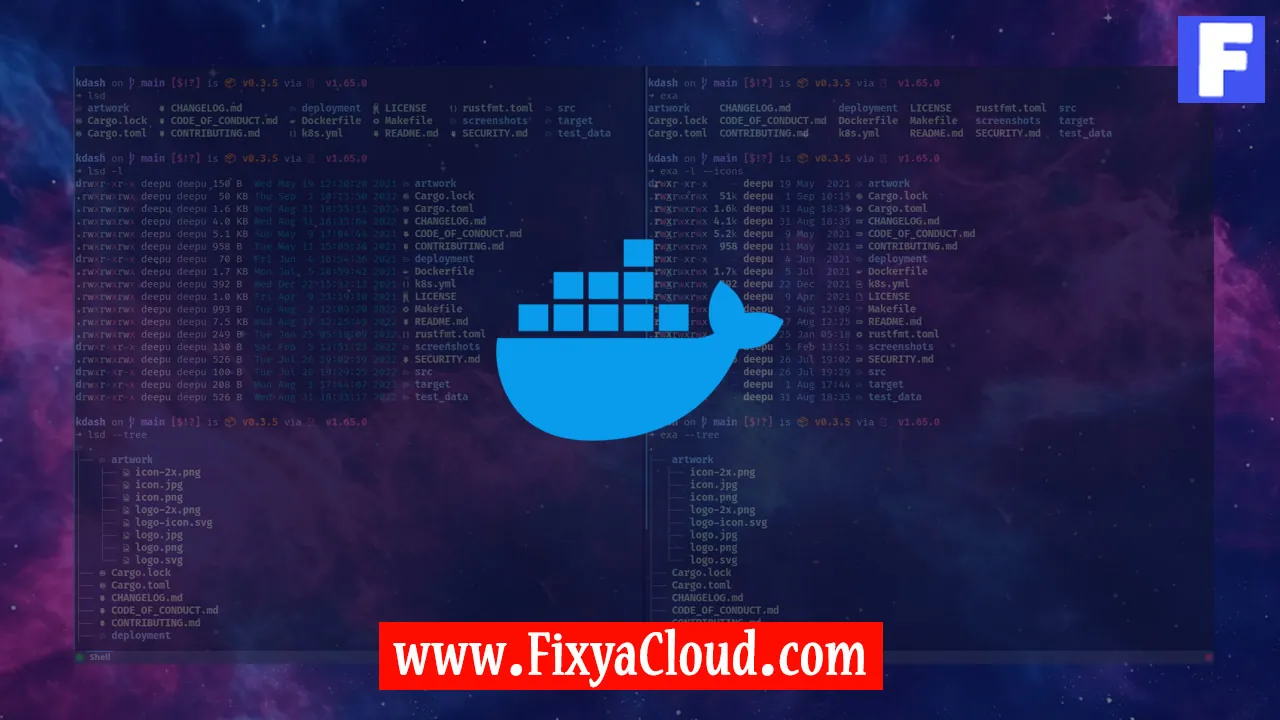
In the dynamic realm of software development, containerization has emerged as a game-changer. Docker, a popular containerization platform, allows developers to encapsulate applications and their dependencies into lightweight, portable containers. This article delves into the process of "Dockerizing" a Java application, providing step-by-step instructions and insightful examples to guide you through the seamless integration of your Java project with Docker.
Prerequisites:
Before embarking on the Dockerization journey, ensure that Docker is installed on your machine. You can download Docker from the official website (https://www.docker.com/). Additionally, make sure you have a Java application ready for containerization.Creating a Dockerfile:
The Dockerfile is the blueprint for your Docker image. Begin by creating a file namedDockerfile
in the root directory of your Java project. Open the Dockerfile in your preferred text editor and start with the following:
# Use an official OpenJDK runtime as a parent image
FROM openjdk:11-jre-slim
# Set the working directory to /app
WORKDIR /app
# Copy the current directory contents into the container at /app
COPY . /app
# Specify the command to run on container start
CMD ["java", "-jar", "your-application.jar"]
Adjust the openjdk
version and the JAR file name according to your project requirements.
- Building the Docker Image:
Open a terminal window and navigate to the directory containing your Dockerfile. Execute the following command to build your Docker image:
docker build -t your-image-name .
Replace your-image-name
with a suitable name for your Docker image.
- Running the Docker Container:
Once the image is built successfully, run the following command to start a Docker container:
docker run -p 8080:8080 your-image-name
This command maps port 8080 on your host machine to port 8080 within the Docker container. Adjust the port numbers as needed.
Accessing the Java Application:
Open your web browser and navigate to http://localhost:8080 to access your Java application running within the Docker container.Additional Docker Commands:
- To list all running containers:
docker ps
- To stop a running container:
docker stop container-id
- To remove a container:
docker rm container-id
- To remove a Docker image:
docker rmi image-id
- Docker Compose for Multi-container Applications:
For more complex applications with multiple services, consider using Docker Compose. Create adocker-compose.yml
file and define the services, networks, and volumes required for your application.
version: '3'
services:
your-app:
build:
context: .
dockerfile: Dockerfile
ports:
- "8080:8080"
Run your multi-container application using:
docker-compose up
More Examples:
- Environment Variables:
You can pass environment variables to your Java application by modifying the Dockerfile:
ENV DATABASE_URL="jdbc:mysql://localhost:3306/mydatabase"
Adjust the environment variables based on your application's configuration.
- Optimizing Docker Images:
Consider using a multi-stage build to optimize your Docker image size. This involves using a separate image for the build phase and copying only the necessary artifacts into the final image.
# Build Stage
FROM maven:3.8.4 AS build
WORKDIR /app
COPY . /app
RUN mvn clean install
# Final Stage
FROM openjdk:11-jre-slim
WORKDIR /app
COPY --from=build /app/target/your-application.jar .
CMD ["java", "-jar", "your-application.jar"]
Dockerizing a Java application streamlines deployment, enhances scalability, and simplifies the management of dependencies. By following these steps and exploring additional examples, you can seamlessly integrate Docker into your Java development workflow. Embrace the power of containerization and elevate your application deployment to new heights.
Related Searches and Questions asked:
That's it for this topic, Hope this article is useful. Thanks for Visiting us.