Dockerize Python Application
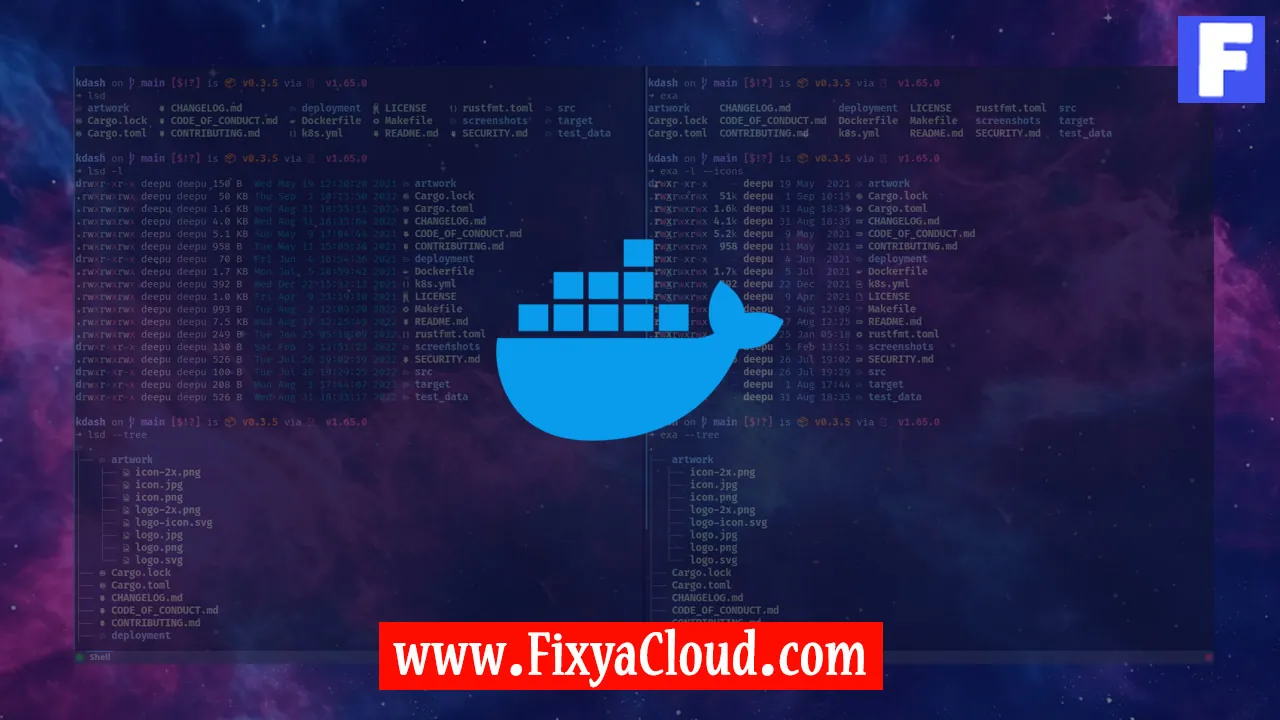
Dockerization has become an essential practice in modern software development, allowing developers to encapsulate their applications and dependencies into lightweight, portable containers. This not only streamlines the deployment process but also ensures consistency across different environments. In this article, we will delve into the process of Dockerizing a Python application, providing step-by-step instructions, useful commands, and additional examples to guide you through the entire process.
Understanding Docker:
Before we embark on Dockerizing a Python application, let's briefly understand what Docker is. Docker is a containerization platform that enables developers to package applications and their dependencies into isolated, portable containers. These containers can run consistently across various environments, eliminating the infamous "it works on my machine" dilemma.Prerequisites:
Ensure you have Docker installed on your machine. You can download the latest version of Docker from the official website (https://www.docker.com/).# Check Docker version
docker --versionSetting Up Your Python Application:
Start by creating a simple Python application or use an existing one. For illustration purposes, let's assume we have a basic Flask web application.# app.py
from flask import Flask
app = Flask(__name__)
@app.route('/')
def hello():
return "Hello, Dockerized Python App!"Creating a Dockerfile:
The Dockerfile is a configuration file that instructs Docker on how to build your application's container. Create a file namedDockerfile
in the project directory and add the following content:# Use an official Python runtime as a parent image
FROM python:3.8-slim
# Set the working directory in the container
WORKDIR /app
# Copy the current directory contents into the container at /app
COPY . /app
# Install any needed packages specified in requirements.txt
RUN pip install --trusted-host pypi.python.org -r requirements.txt
# Make port 80 available to the world outside this container
EXPOSE 80
# Define environment variable
ENV NAME World
# Run app.py when the container launches
CMD ["python", "app.py"]Building the Docker Image:
Build the Docker image using the following command:docker build -t my-python-app .
Running the Docker Container:
Once the image is built, run a container from it:docker run -p 4000:80 my-python-app
Open your browser and navigate to http://localhost:4000 to see your Dockerized Python application in action.
Additional Docker Commands:
List all running containers:
docker ps
Stop a running container:
docker stop <container_id>
Remove a container:
docker rm <container_id>
View container logs:
docker logs <container_id>
Dockerizing a Python application enhances portability, scalability, and consistency in deployment. By following these steps and commands, you've successfully containerized your Python application, paving the way for more efficient and reliable software development.
Related Searches and Questions asked:
That's it for this topic, Hope this article is useful. Thanks for Visiting us.