How to Deploy a Python Application on Docker
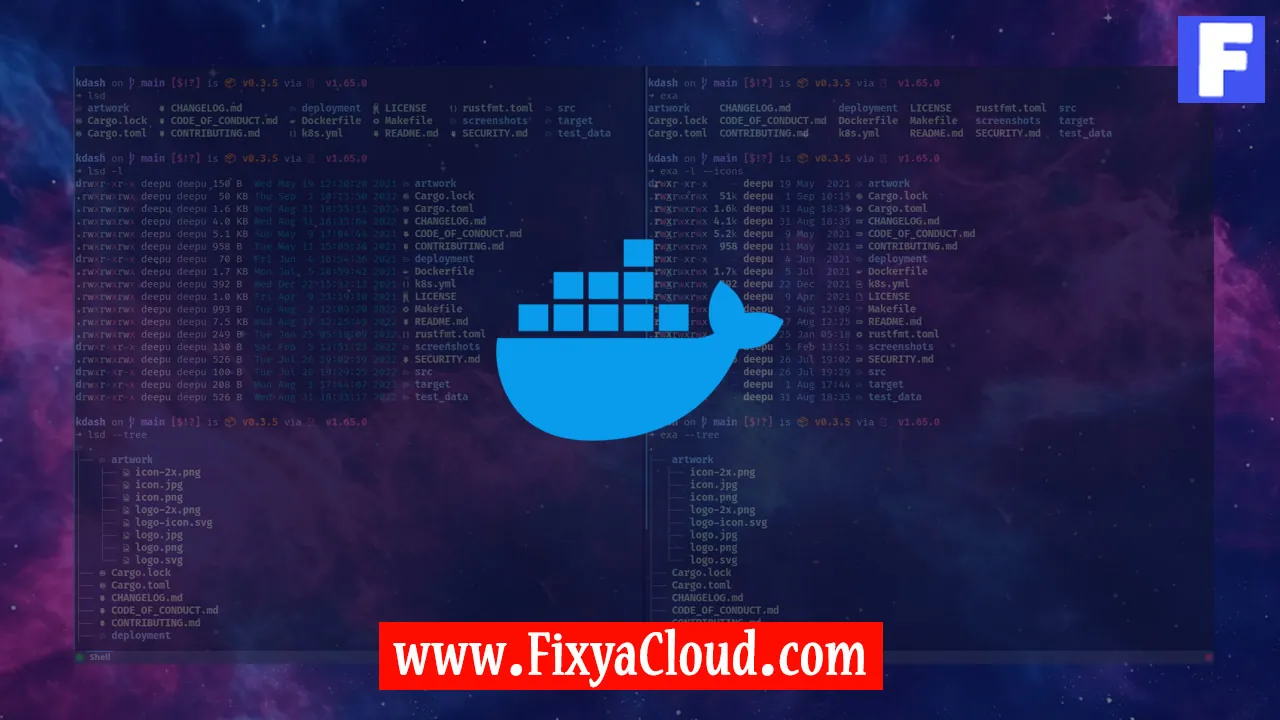
In the ever-evolving landscape of software development, containerization has become a cornerstone technology for deploying applications seamlessly across different environments. Docker, a popular containerization platform, offers a streamlined approach to packaging, distributing, and running applications in isolated containers. In this guide, we will explore the step-by-step process of deploying a Python application using Docker, enabling you to encapsulate your application and its dependencies into a portable and reproducible container.
Getting Started: Installing Docker
Before we delve into deploying our Python application, let's ensure Docker is installed on your machine. If you haven't installed Docker yet, you can download it from the official website: Docker Official Website.
Creating a Python Application:
For the purpose of this guide, let's assume we have a simple Flask web application. Create a directory for your project and navigate into it.
mkdir my_python_app
cd my_python_app
Writing the Dockerfile:
Create a file named Dockerfile
in your project directory. The Dockerfile defines the steps to build your Docker image.
# Use an official Python runtime as a parent image
FROM python:3.8-slim
# Set the working directory to /app
WORKDIR /app
# Copy the current directory contents into the container at /app
COPY . /app
# Install any needed packages specified in requirements.txt
RUN pip install --trusted-host pypi.python.org -r requirements.txt
# Make port 80 available to the world outside this container
EXPOSE 80
# Define environment variable
ENV NAME World
# Run app.py when the container launches
CMD ["python", "app.py"]
Make sure to replace app.py
and requirements.txt
with the appropriate names for your Python application.
Building the Docker Image:
In your project directory, execute the following command to build your Docker image.
docker build -t my_python_app .
This command tells Docker to build an image from the current directory (.
) and tag it with the name my_python_app
.
Running the Docker Container:
Once the image is built, you can run your Docker container using the following command:
docker run -p 4000:80 my_python_app
This command maps port 4000 on your host machine to port 80 on the Docker container.
Accessing Your Python Application:
Open your web browser and navigate to http://localhost:4000
. You should see your Python application running inside a Docker container.
Additional Docker Commands:
- To list running containers:
docker ps
- To stop a running container:
docker stop <container_id>
- To remove a container:
docker rm <container_id>
- To remove an image:
docker rmi <image_id>
Congratulations! You've successfully deployed a Python application using Docker. Containerization provides a convenient and consistent way to package and run applications across various environments. Experiment with Docker, explore its features, and adapt it to suit your specific use cases.
Related Searches and Questions asked:
That's it for this topic, Hope this article is useful. Thanks for Visiting us.