How to Build Python Application With Docker
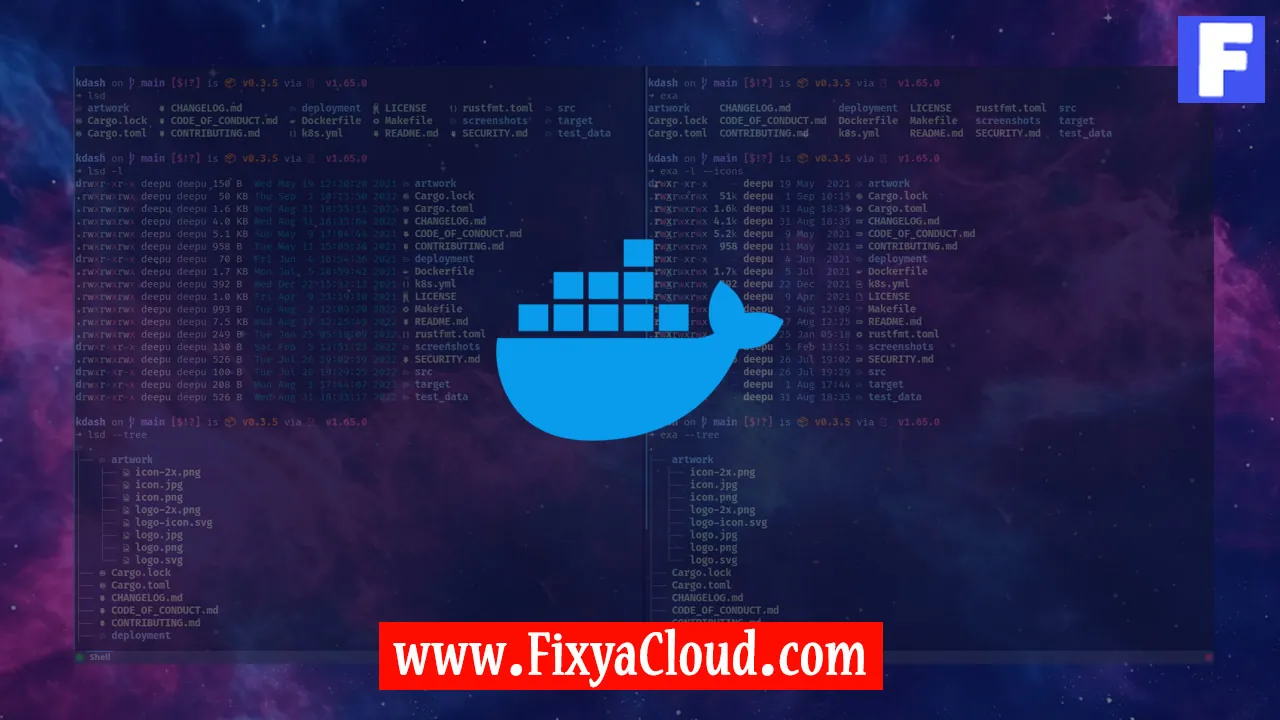
Docker has revolutionized the way we develop, deploy, and manage applications by providing a platform for containerization. Python, being a versatile and widely used programming language, seamlessly integrates with Docker to simplify the application development and deployment process. In this article, we will guide you through the steps of building a Python application with Docker, enabling you to harness the power of containerization for your projects.
Setting Up Your Environment
Before we dive into the process of building a Python application with Docker, ensure you have Docker installed on your system. You can download and install Docker from the official website (https://www.docker.com/get-started).
Writing Your Python Application
Create a simple Python application for demonstration purposes. Let's name it app.py
:
# app.py
print("Hello, Dockerized Python Application!")
Dockerfile: Defining the Container
To containerize your Python application, you need a Dockerfile. Create a file named Dockerfile
in the same directory as your Python script and add the following content:
# Use an official Python runtime as a parent image
FROM python:3.9-slim
# Set the working directory in the container
WORKDIR /app
# Copy the current directory contents into the container at /app
COPY . /app
# Install any needed packages specified in requirements.txt
RUN pip install --no-cache-dir -r requirements.txt
# Run app.py when the container launches
CMD ["python", "app.py"]
Building the Docker Image
Open a terminal and navigate to the directory containing your Python script and Dockerfile. Run the following command to build the Docker image:
docker build -t python-docker-app .
Running the Docker Container
Once the image is built, you can run the Docker container using the following command:
docker run python-docker-app
Docker Compose for Multi-Container Applications
For more complex applications with multiple services, consider using Docker Compose. Create a docker-compose.yml
file:
version: '3'
services:
python-app:
build: .
ports:
- "5000:5000"
Run the application using Docker Compose:
docker-compose up
Additional Considerations
- Managing Dependencies: If your Python application has dependencies, ensure they are listed in a
requirements.txt
file. - Environment Variables: Utilize environment variables in your Dockerfile for configurations that may vary between environments.
In this article, we covered the fundamental steps to build a Python application with Docker. From setting up your environment to writing a Dockerfile and running containers, you now have a solid foundation for containerizing your Python projects. Docker's versatility and scalability make it an invaluable tool for modern software development.
Related Searches and Questions asked:
That's it for this topic, Hope this article is useful. Thanks for Visiting us.