How to Run Python on Docker
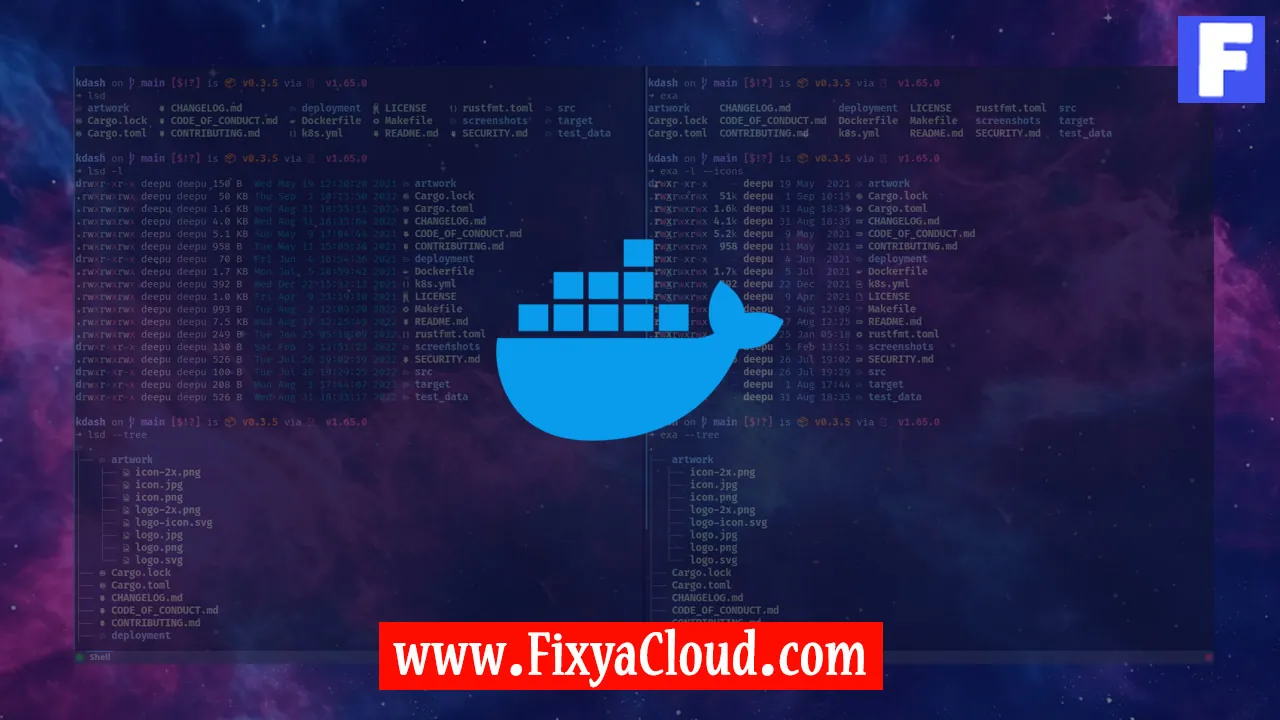
Running Python applications on Docker provides a convenient and efficient way to manage dependencies, isolate environments, and streamline the deployment process. Docker containers encapsulate the application and its dependencies, ensuring consistency across different environments. In this guide, we will walk through the steps of running Python applications on Docker, covering essential commands, best practices, and practical examples.
Setting Up Docker:
Before diving into running Python on Docker, make sure you have Docker installed on your machine. Visit the official Docker website (https://www.docker.com/get-started) to download and install Docker for your operating system.
Creating a Dockerfile:
The first step in running Python on Docker is creating a Dockerfile. This file contains instructions for building a Docker image, which is a lightweight, standalone, and executable package that includes the application code and its dependencies. Here's a simple example of a Dockerfile:
# Use an official Python runtime as a parent image
FROM python:3.9
# Set the working directory in the container
WORKDIR /app
# Copy the current directory contents into the container at /app
COPY . /app
# Install any needed packages specified in requirements.txt
RUN pip install --no-cache-dir -r requirements.txt
# Define environment variable
ENV NAME World
# Run app.py when the container launches
CMD ["python", "app.py"]
This Dockerfile uses the official Python 3.9 image, sets the working directory, copies the application code, installs dependencies from the requirements.txt
file, sets an environment variable, and specifies the default command to run the application.
Building the Docker Image:
Navigate to the directory containing your Dockerfile and run the following command to build the Docker image:
docker build -t my-python-app .
This command tags the image with the name "my-python-app," and the dot at the end signifies the build context as the current directory.
Running the Docker Container:
Once the image is built, you can run a Docker container based on that image. Use the following command:
docker run -it --rm my-python-app
The -it
flag allows interactive mode, and the --rm
flag removes the container when it exits. Replace "my-python-app" with the name you provided during the image build.
Additional Commands and Tips:
- To list all running containers, use:
docker ps
- To access the shell of a running container, use:
docker exec -it <container_id_or_name> /bin/bash
Running Python on Docker is a powerful strategy for maintaining consistency and simplicity in your development and deployment processes. By encapsulating your application and its dependencies, Docker provides a standardized and reproducible environment across different systems. Whether you're working on a small project or a large-scale application, incorporating Docker into your workflow can greatly enhance efficiency and ease of deployment.
Related Searches and Questions asked:
That's it for this topic, Hope this article is useful. Thanks for Visiting us.