Python is buffering its stdout in AWS EKS
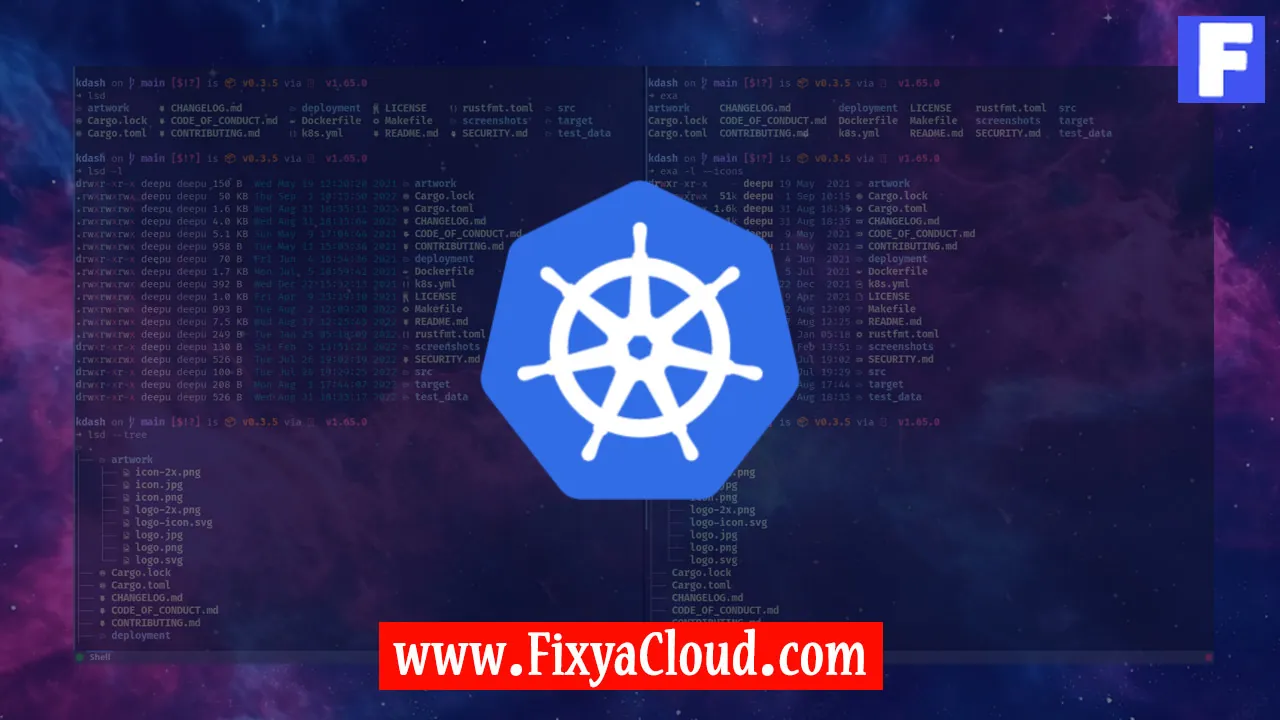
Python developers working with AWS EKS (Elastic Kubernetes Service) may encounter a peculiar issue where the standard output (stdout) appears to be buffered. This phenomenon can lead to delayed or out-of-sequence log messages, making debugging and troubleshooting more challenging. In this article, we'll delve into the reasons behind Python buffering its stdout in AWS EKS and explore solutions to address this issue effectively.
Understanding the Buffering Issue:
When running Python applications in AWS EKS, developers may observe that the output generated using print()
statements or any other method doesn't appear immediately in the logs. Instead, it seems to be buffered, causing delays in the display of log messages.
Possible Causes:
Several factors contribute to this buffering behavior, including the environment in which the Python application is running and the specific configurations of AWS EKS. To tackle this issue, let's explore potential causes and corresponding solutions.
1. Containerization Impact:
Containers in AWS EKS may introduce additional layers of buffering. To mitigate this, consider adjusting the buffering settings for Python.
# Disable output buffering
print("Hello, World!", flush=True)
2. EKS Logging Configuration:
Review the logging configurations in your EKS cluster. Ensure that there are no conflicting settings that might interfere with the real-time display of Python stdout.
# Check EKS cluster logs
kubectl logs <pod_name>
3. Lambda Function Considerations:
If you are deploying Python code as AWS Lambda functions within EKS, check for Lambda-specific considerations that might impact stdout behavior.
# Adjust Lambda-specific buffering settings
import sys
sys.stdout = sys.stderr # Disable buffering
Step-by-Step Instructions:
Identify the Pod:
Use the following command to list all the pods in your EKS cluster and identify the pod running your Python application.kubectl get pods
Inspect Logging Configuration:
Examine the logging configuration of your EKS cluster to ensure there are no conflicting settings that may cause stdout buffering.kubectl describe configmap <config_map_name>
Container Logs:
Check the logs of your Python container to see if there are any messages related to buffering or delayed stdout.kubectl logs <pod_name>
Adjust Python Code:
Modify your Python code to disable buffering explicitly. This can be done using theflush=True
argument with theprint()
function.print("Hello, World!", flush=True)
Additional Examples:
Here are additional examples illustrating how to handle buffering in different scenarios:
File Redirection:
If you are redirecting stdout to a file, ensure that the file is set to unbuffered mode.python script.py > output.log
AWS Lambda Function:
When deploying Python code as an AWS Lambda function within EKS, consider the following adjustments:import sys
sys.stdout = sys.stderr # Disable buffering
So, addressing Python stdout buffering in AWS EKS involves a combination of understanding containerization impacts, checking EKS logging configurations, and making adjustments in your Python code. By following the steps and examples provided, you can enhance the real-time visibility of your application's output.
Related Searches and Questions asked:
That's it for this topic, Hope this article is useful. Thanks for Visiting us.