Containerize Java Best Practices
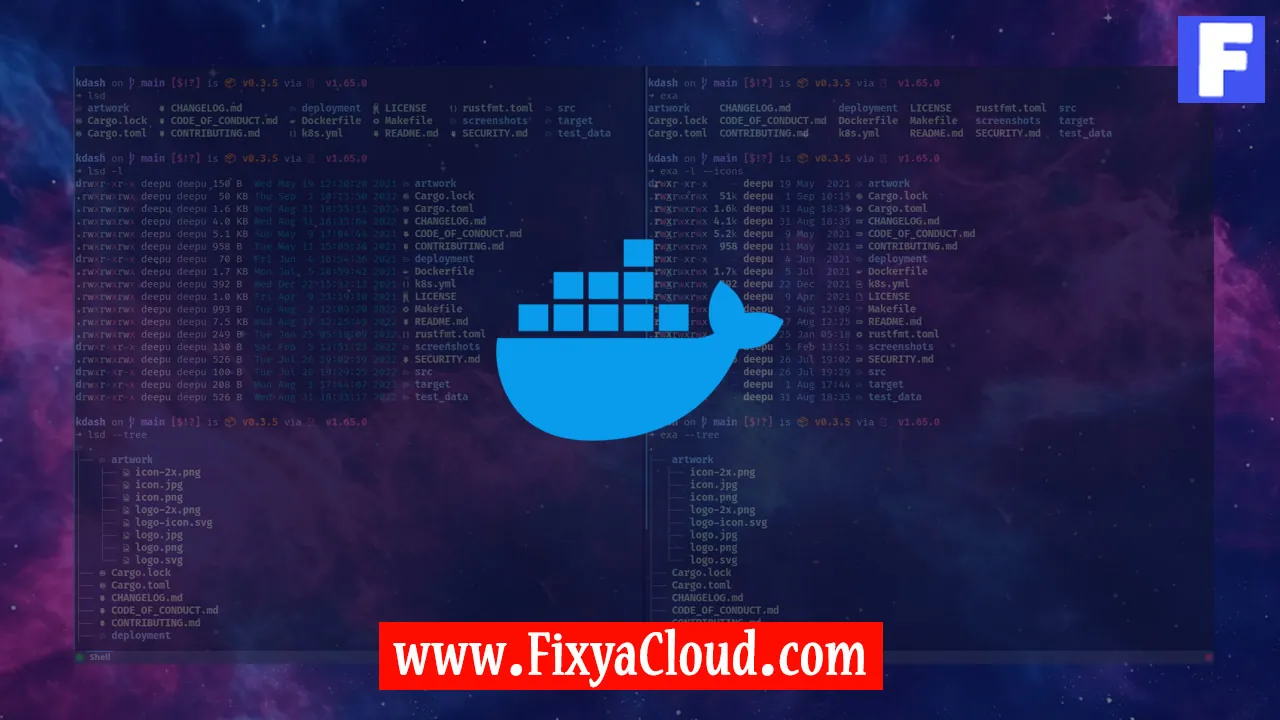
In the ever-evolving landscape of software development, containerization has become a pivotal technology. It allows developers to encapsulate applications and their dependencies, ensuring consistency across different environments. Java, being one of the most widely used programming languages, can benefit significantly from containerization. In this article, we will delve into the best practices for containerizing Java applications, guiding you through the process step by step.
1. Choose the Right Base Image:
Selecting an appropriate base image is crucial for building efficient and secure containers. For Java applications, using an official OpenJDK or AdoptOpenJDK image is recommended. These images come with a minimal footprint and are regularly updated with security patches.
# Example Dockerfile snippet for a Java 11 application
FROM adoptopenjdk:11-jre-hotspot
2. Optimize Your Docker Image:
To minimize the container size and enhance performance, consider using multi-stage builds. This involves creating multiple Docker images, with each stage serving a specific purpose. The final image only includes the necessary artifacts, resulting in a lean and efficient container.
# Example multi-stage Dockerfile
FROM maven:3.8.1-jdk-11 AS builder
WORKDIR /app
COPY . .
RUN mvn package
FROM adoptopenjdk:11-jre-hotspot
WORKDIR /app
COPY --from=builder /app/target/myapp.jar .
CMD ["java", "-jar", "myapp.jar"]
3. Leverage Container Orchestration:
When deploying Java applications at scale, container orchestration tools like Kubernetes can simplify management. They enable automated scaling, rolling updates, and load balancing. Ensure your application is designed to work seamlessly with these orchestration platforms.
# Example Kubernetes Deployment manifest
apiVersion: apps/v1
kind: Deployment
metadata:
name: myapp
spec:
replicas: 3
selector:
matchLabels:
app: myapp
template:
metadata:
labels:
app: myapp
spec:
containers:
- name: myapp
image: myapp:latest
ports:
- containerPort: 8080
4. Manage Environment Variables Securely:
Handle sensitive information, such as database credentials or API keys, securely in your Java applications. Use environment variables and avoid hardcoding these values in your code or configuration files. Docker allows you to pass environment variables easily during container runtime.
# Example Docker run command with environment variables
docker run -e DATABASE_URL=jdbc:mysql://localhost:3306/mydb -e DB_USERNAME=user -e DB_PASSWORD=secret myapp:latest
5. Monitor and Troubleshoot:
Implementing proper monitoring is essential for identifying issues and optimizing Java containers. Use tools like Prometheus and Grafana to collect and visualize metrics. Additionally, include logging mechanisms in your application to aid in troubleshooting.
6. Regularly Update Dependencies:
Keep your Java dependencies, including libraries and frameworks, up to date to benefit from the latest features and security patches. Regularly check for updates and incorporate them into your containerized Java application.
Related Searches and Questions asked:
That's it for this topic, Hope this article is useful. Thanks for Visiting us.