How to Run Java App on Docker
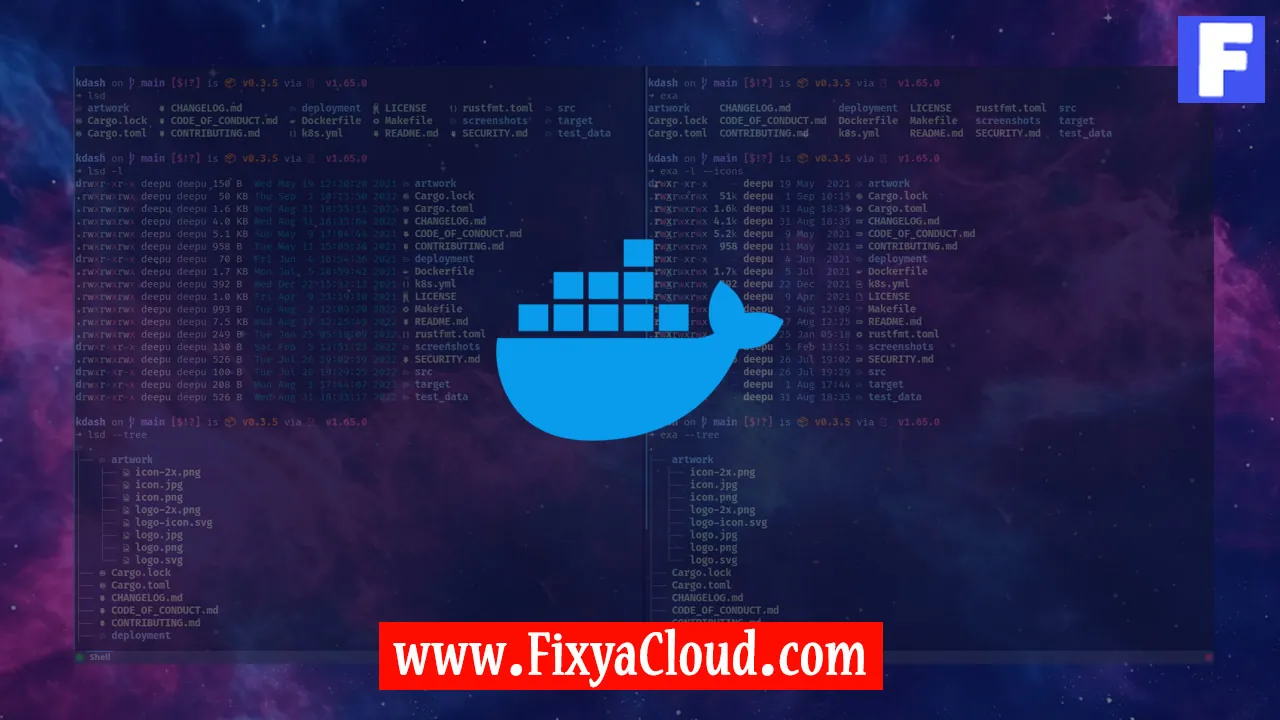
Running a Java application on Docker provides numerous benefits, including portability, scalability, and ease of deployment. Docker containers encapsulate all dependencies, making it easier to manage and ship applications across various environments. In this guide, we'll walk you through the process of running a Java application on Docker, breaking down the steps to ensure a smooth deployment.
Setting Up Your Environment:
Before diving into Dockerizing your Java app, make sure you have Docker installed on your machine. You can download and install Docker from the official website (https://www.docker.com/get-started).
Creating a Simple Java Application:
To demonstrate the process, let's start with a basic Java application. Create a new directory for your project and add a simple Java class, for example, HelloWorld.java
:
public class HelloWorld {
public static void main(String[] args) {
System.out.println("Hello, Docker!");
}
}
Building the Java Application:
Compile your Java application using the following command:
javac HelloWorld.java
This command will generate a HelloWorld.class
file.
Writing a Dockerfile:
Next, create a Dockerfile
in the same directory as your Java application. This file contains instructions for building a Docker image for your Java app:
# Use an official OpenJDK runtime as a parent image
FROM openjdk:11-jre-slim
# Set the working directory in the container
WORKDIR /usr/src/app
# Copy the application JAR file into the container
COPY HelloWorld.class .
# Run the application when the container launches
CMD ["java", "HelloWorld"]
Building the Docker Image:
Build the Docker image using the following command:
docker build -t my-java-app .
Running the Docker Container:
Once the image is built, run the Docker container:
docker run my-java-app
You should see the "Hello, Docker!" message printed in the console, indicating that your Java application is running inside the Docker container.
Additional Commands and Considerations:
View Running Containers:
To view the list of running containers, use:
docker ps
Stop a Running Container:
If you need to stop a running container, use the following command:
docker stop <container_id>
Remove a Container:
To remove a container, use:
docker rm <container_id>
Congratulations! You have successfully Dockerized a simple Java application. This process can be extended to more complex Java projects, allowing you to leverage the power of Docker for efficient application deployment.
Related Searches and Questions asked:
That's it for this topic, Hope this article is useful. Thanks for Visiting us.