Containerize Spring Boot Best Practices
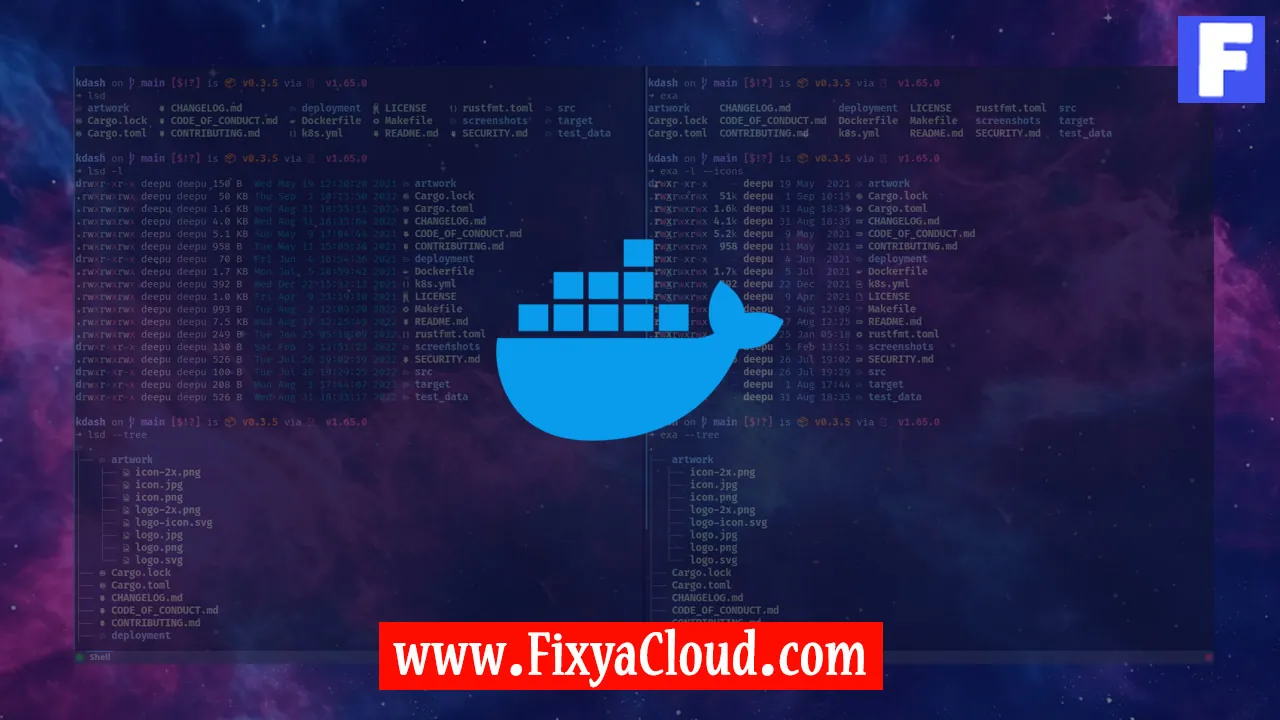
In the ever-evolving landscape of software development, containerization has become a cornerstone for deploying and managing applications efficiently. Spring Boot, a popular Java-based framework for building microservices, can greatly benefit from containerization. This article explores the best practices for containerizing Spring Boot applications, guiding you through essential steps to ensure a seamless and efficient deployment process.
Understanding Containerization and Spring Boot Integration:
Before delving into best practices, let's briefly understand the synergy between containerization and Spring Boot. Containers encapsulate an application and its dependencies, providing a consistent environment across different deployment stages. Integrating Spring Boot with containers enables developers to create portable, scalable, and easily managed applications.Selecting the Right Base Image:
Begin the containerization process by selecting an appropriate base image. Choosing a lightweight and secure base image is crucial for optimizing the size and security of your container. Popular choices include OpenJDK-based images or Alpine Linux images.Leveraging Multi-Stage Builds:
Optimize your Dockerfile by employing multi-stage builds. This technique involves using multiple build stages to reduce the size of the final container image. This not only enhances security but also minimizes the attack surface by excluding unnecessary build artifacts from the production image.
Commands:
# Example of a multi-stage Dockerfile for Spring Boot
# Build Stage
FROM maven:3.8.4-openjdk-17 AS build
COPY . /app
WORKDIR /app
RUN mvn clean package
# Production Stage
FROM openjdk:17-alpine
COPY --from=build /app/target/my-spring-app.jar /app.jar
CMD ["java", "-jar", "/app.jar"]
- Optimizing Jar Packaging:
When packaging your Spring Boot application into a JAR file, consider using therepackage
goal. This goal optimizes the JAR file for execution within a containerized environment, ensuring that all dependencies are included.
# Example Maven command for repackaging
mvn clean package spring-boot:repackage
Step-by-Step Instructions:
- First, ensure that you have Docker installed on your development machine.
- Create a Dockerfile in the root directory of your Spring Boot project.
- Select a suitable base image, considering factors like size and security.
- Implement multi-stage builds in your Dockerfile to separate build and production stages.
- Leverage the
spring-boot:repackage
goal during the Maven build process to optimize JAR packaging. - Build your Docker image using the
docker build
command. - Run the containerized Spring Boot application locally to verify its functionality.
More Examples:
To illustrate the power of containerization for Spring Boot, let's consider a real-world example. Suppose you have a microservices architecture, and each microservice is a separate Spring Boot application. By containerizing each microservice, you can easily deploy, scale, and manage the entire ecosystem with tools like Kubernetes.
Additionally, consider integrating container orchestration tools such as Kubernetes for deploying and managing your containerized Spring Boot applications in a production environment. Kubernetes provides features like auto-scaling, load balancing, and rolling updates, ensuring high availability and seamless deployments.
Containerizing Spring Boot applications is a best practice that brings numerous benefits, from improved portability to enhanced security and scalability. By following the outlined best practices, you can ensure a smooth and efficient containerization process for your Spring Boot projects. Embrace containerization to stay ahead in the dynamic world of software development.
Related Searches and Questions asked:
That's it for this topic, Hope this article is useful. Thanks for Visiting us.