Containerize Python Best Practices
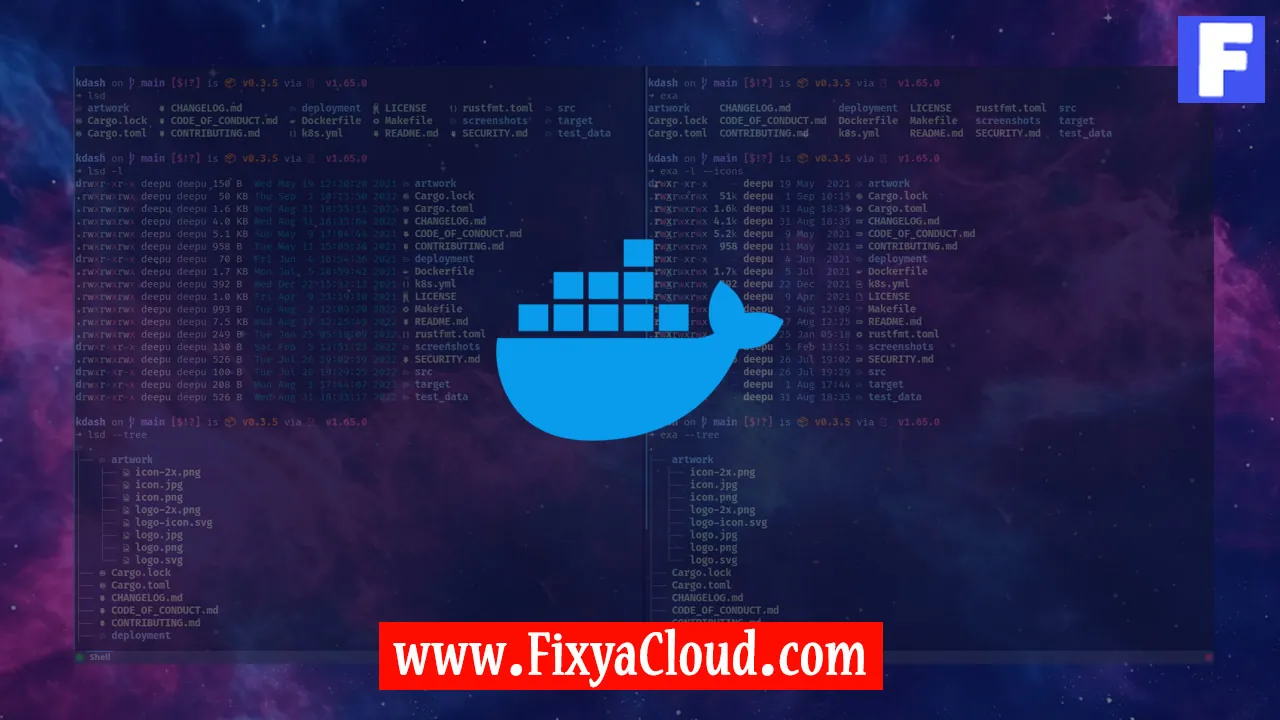
In the ever-evolving landscape of software development, containerization has emerged as a transformative technology, allowing developers to encapsulate their applications along with their dependencies, ensuring consistency and portability across different environments. Python, being a versatile and widely-used programming language, can greatly benefit from containerization. In this article, we will explore the best practices for containerizing Python applications, from choosing the right base image to optimizing the Dockerfile and managing dependencies.
- Choosing the Right Base Image:
Selecting an appropriate base image is a crucial first step in containerizing Python applications. Consider using official Python images from Docker Hub, as they are regularly updated and maintained. Choose the version of Python that aligns with your application requirements, balancing the need for new features with stability.
# Use an official Python runtime as a parent image
FROM python:3.8-slim
- Managing Dependencies:
Efficiently managing dependencies is essential to ensure reproducibility and consistency across different environments. Utilize a virtual environment within your Docker container to isolate dependencies.
# Create and set up a virtual environment
RUN python -m venv /opt/venv
ENV PATH="/opt/venv/bin:$PATH"
# Copy and install dependencies
COPY requirements.txt /app/
RUN pip install --no-cache-dir -r /app/requirements.txt
- Optimizing the Dockerfile:
Keep your Dockerfile simple and minimize layers to reduce image size. Use multi-stage builds to separate build dependencies from the final image, resulting in a smaller footprint.
# Use a multi-stage build for smaller final image
FROM python:3.8-slim AS builder
WORKDIR /app
COPY requirements.txt .
RUN python -m venv /opt/venv
ENV PATH="/opt/venv/bin:$PATH"
RUN pip install --no-cache-dir -r requirements.txt
FROM python:3.8-slim
WORKDIR /app
COPY --from=builder /opt/venv /opt/venv
ENV PATH="/opt/venv/bin:$PATH"
COPY . .
- Handling Application Code:
Copy only necessary files into the container to minimize the build context. This helps in reducing the time and resources required for building the image.
# Copy only necessary files
COPY src/ /app/src
COPY main.py /app/main.py
- Running as Non-Root User:
For security reasons, it's a good practice to run your Python application using a non-root user. Create a dedicated user and switch to it before running the application.
# Create a non-root user
RUN useradd -r -u 1001 appuser
USER appuser
# Command to run the application
CMD ["python", "main.py"]
- Docker Compose for Local Development:
Simplify local development by using Docker Compose to define and run multi-container Docker applications. This allows you to specify all the services, networks, and volumes in a single YAML file.
version: '3'
services:
myapp:
build:
context: .
ports:
- "5000:5000"
- Utilizing Environment Variables:
Use environment variables to parameterize your application and make it more configurable. This allows for easy customization without modifying the Docker image.
# Set environment variable for Flask app
ENV FLASK_APP=main.py
CMD ["flask", "run", "--host=0.0.0.0"]
So, containerizing Python applications brings numerous advantages in terms of reproducibility, scalability, and ease of deployment. By following these best practices, you can ensure your Python containers are efficient, secure, and well-optimized.
Related Searches and Questions asked:
That's it for this topic, Hope this article is useful. Thanks for Visiting us.