Deploy Java Application on Docker
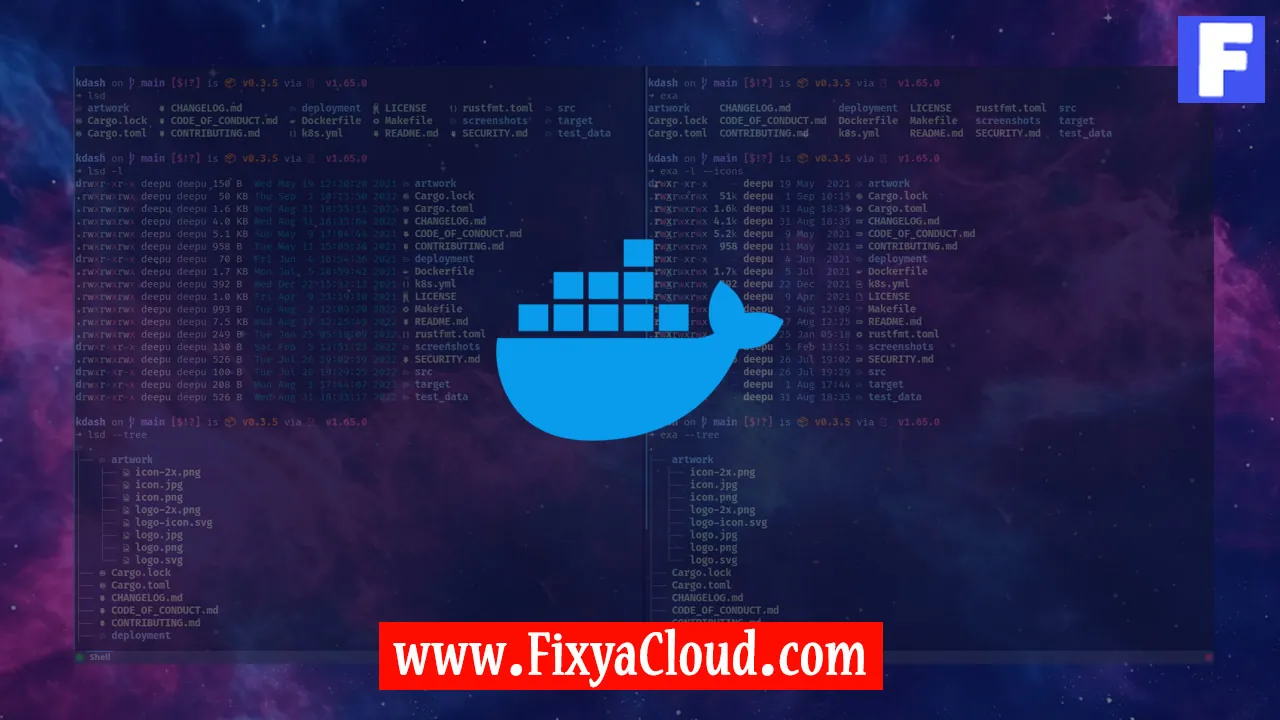
In the ever-evolving landscape of software development, deploying applications efficiently is crucial. Docker has emerged as a powerful tool for containerization, providing a consistent environment across different stages of development and deployment. In this article, we will explore the process of deploying a Java application on Docker, step by step.
Setting Up the Environment:
Before diving into the deployment process, ensure that you have Docker installed on your machine. You can download Docker from the official website (https://www.docker.com/products/docker-desktop) and follow the installation instructions provided.
Creating a Simple Java Application:
Let's start by creating a basic Java application. For this example, we'll create a "HelloWorld" application. Open your favorite text editor and create a file named HelloWorld.java
with the following content:
public class HelloWorld {
public static void main(String[] args) {
System.out.println("Hello, Docker!");
}
}
Save the file and compile it using the following command:
javac HelloWorld.java
Writing a Dockerfile:
To containerize our Java application, we need to create a Dockerfile. This file contains instructions for building a Docker image. Create a file named Dockerfile
in the same directory as your Java file with the following content:
# Use an official OpenJDK runtime as a parent image
FROM openjdk:11-jre-slim
# Set the working directory
WORKDIR /usr/src/app
# Copy the application JAR file into the container
COPY HelloWorld.class .
# Run the application when the container starts
CMD ["java", "HelloWorld"]
Building the Docker Image:
Now, it's time to build the Docker image. Open a terminal in the same directory as your Dockerfile and run the following command:
docker build -t my-java-app .
This command builds a Docker image named my-java-app
using the instructions in your Dockerfile.
Running the Docker Container:
Once the image is built, you can run a container using the following command:
docker run my-java-app
Congratulations! You have successfully deployed a Java application inside a Docker container. You can customize this process for more complex applications by modifying the Dockerfile and adapting the commands accordingly.
Additional Docker Commands:
Here are some additional Docker commands that might come in handy:
List all running containers:
docker ps
Stop a running container:
docker stop <container_id>
Remove a container:
docker rm <container_id>
Remove an image:
docker rmi <image_id>
Docker simplifies the deployment process, offering consistency and scalability. By containerizing your Java applications, you ensure they run seamlessly across different environments. Experiment with different configurations and explore Docker's vast ecosystem to enhance your deployment workflows.
Related Searches and Questions asked:
That's it for this topic, Hope this article is useful. Thanks for Visiting us.