Containerize NodeJS Best Practices
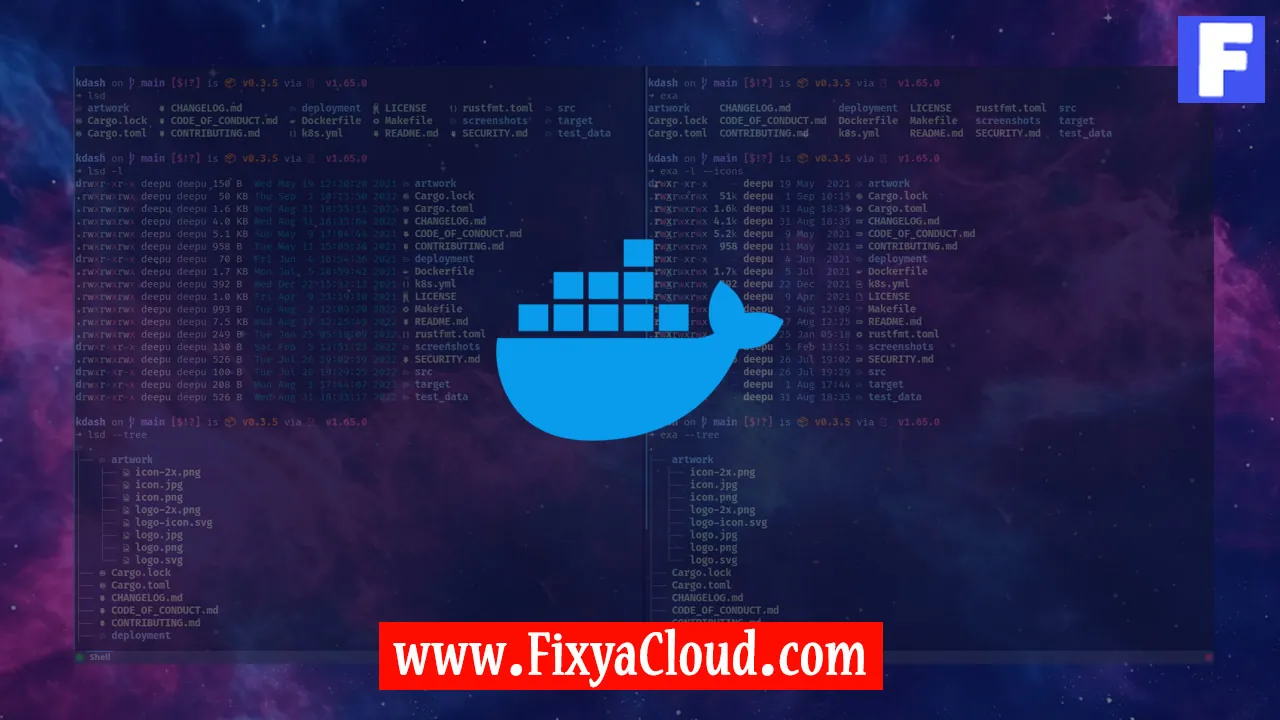
In the dynamic landscape of modern software development, containerization has become a cornerstone for building scalable and portable applications. Node.js, with its lightweight and event-driven architecture, is a popular choice for developers. Containerizing Node.js applications not only ensures consistency across different environments but also streamlines deployment processes. In this article, we will explore the best practices for containerizing Node.js applications, providing you with a comprehensive guide to enhance your development workflow.
Choosing the Right Base Image:
When containerizing a Node.js application, selecting an appropriate base image is crucial. Choose a lightweight and secure base image to minimize the container size and reduce potential security vulnerabilities. A popular choice is the official Node.js Docker image, which provides a solid foundation for running Node.js applications.FROM node:14-alpine
Optimizing Dockerfile:
Craft a Dockerfile that optimally sets up your Node.js application within the container. Leverage Docker layers to cache dependencies and improve build times. Prioritize installing dependencies before copying application code to take advantage of Docker's layer caching mechanism.# Install dependencies
COPY package*.json ./
RUN npm install
# Copy application code
COPY . .
# Expose the application port
EXPOSE 3000
# Command to run the application
CMD ["npm", "start"]Environment Variables:
Utilize environment variables to make your Node.js application configurable. This practice ensures flexibility and makes it easy to configure the application's behavior without modifying the code. Docker allows you to pass environment variables during container runtime.# Set environment variables
ENV NODE_ENV=production
ENV PORT=3000Health Checks:
Implement health checks in your Dockerfile to enhance container orchestration. This ensures that the container is healthy and ready to handle requests. Define a health check endpoint in your Node.js application to let Docker monitor the application's status.HEALTHCHECK --interval=30s CMD curl -fs http://localhost:${PORT}/health || exit 1
Note: Adjust the health check endpoint based on your application.
Multi-Stage Builds:
Employ multi-stage builds to reduce the size of your final Docker image. This involves using separate Docker images for building and running the application. The final image only contains the necessary artifacts, resulting in a smaller footprint.# Build stage
FROM node:14-alpine as build
WORKDIR /app
COPY . .
RUN npm install
RUN npm run build
# Run stage
FROM node:14-alpine
WORKDIR /app
COPY --from=build /app/dist ./dist
CMD ["npm", "start"]
Related Searches and Questions asked:
That's it for this topic, Hope this article is useful. Thanks for Visiting us.